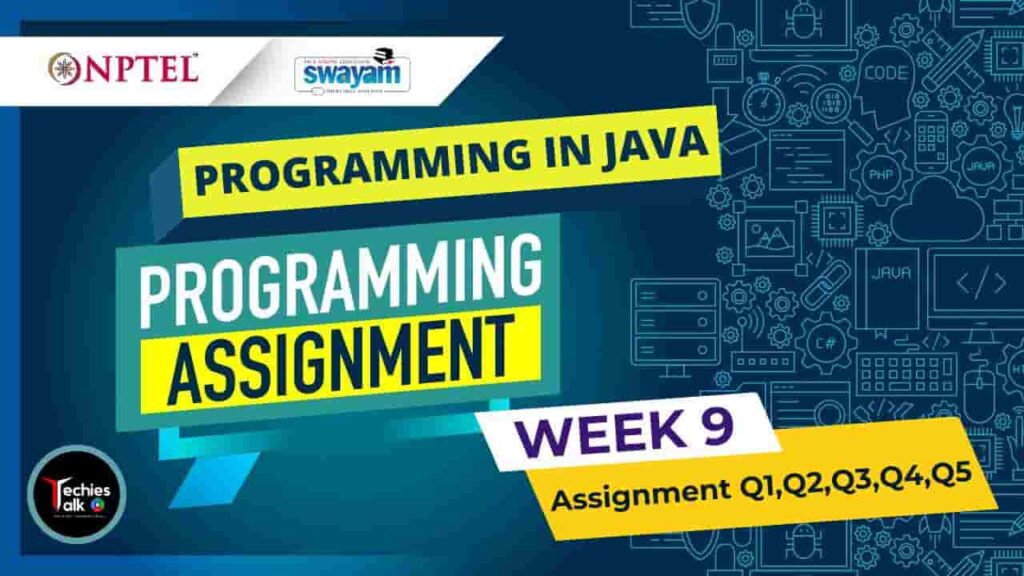
NPTEL Programming in Java Week 9 All Programming Assignment Solutions – January 2023 | Swayam. With the growth of Information and Communication Technology, there is a need to develop large and complex software.
Further, those software should be platform independent, Internet enabled, easy to modify, secure, and robust. To meet this requirement object-oriented paradigm has been developed and based on this paradigm the Java programming language emerges as the best programming environment.
Now, Java programming language is being used for mobile programming, Internet programming, and many other applications compatible to distributed systems.
This course aims to cover the essential topics of Java programming so that the participants can improve their skills to cope with the current demand of IT industries and solve many problems in their own filed of studies.
COURSE LAYOUT
- Week 1 : Overview of Object-Oriented Programming and Java
- Week 2 : Java Programming Elements
- Week 3 : Input-Output Handling in Java
- Week 4 : Encapsulation
- Week 5 : Inheritance
- Week 6 : Exception Handling
- Week 7 : Multithreaded Programming
- Week 8 : Java Applets and Servlets
- Week 9 : Java Swing and Abstract Windowing Toolkit (AWT)
- Week 10 : Networking with Java
- Week 11: Java Object Database Connectivity (ODBC)
- Week 12: Interface and Packages for Software Development
Course Name : “Programming in Java 2023”
Question : 1 Write suitable code to develop a 2D Flip-Flop Array with dimension 5 × 5, which replaces all input elements with values 0 by 1 and 1 by 0. An example is shown below:
INPUT:
00001
00001
00001
00001
00001
OUTPUT:
11110
11110
11110
11110
11110
import java.util.Scanner;
public class Question95{
public static void main(String args[]){
Scanner sc = new Scanner(System.in);
// Declare the 5X5 2D array to store the input
char original[][]= new char[5][5];
// Input 2D Array using Scanner Class and check data validity
for(int line=0;line<5; line++){
String input = sc.nextLine();
char seq[] = input.toCharArray();
if(seq.length==5){
for(int i=0;i<5;i++){
if(seq[i]=='0' || seq[i]=='1'){
original[line][i]=seq[i];
if(line==4 && i==4)
flipflop(original);
}
else{
System.out.print("Only 0 and 1 supported.");
break;
}
}
}else{
System.out.print("Invalid length");
break;
}
}
}
static void flipflop(char[][] flip){
// Flip-Flop Operation
for(int i=0; i<5;i++){
for(int j=0; j<5;j++){
if(flip[i][j]=='1')
flip[i][j]='0';
else
flip[i][j]='1';
}
}
// Output the 2D FlipFlopped Array
for(int i=0; i<5;i++){
for(int j=0; j<5;j++){
System.out.print(flip[i][j]);
}
System.out.println();
}
}
}
Course Name : “Programming in Java 2023”
Question : 2 A program needs to be developed which can mirror reflect any 5 × 5 2D character array into its side-by-side reflection. Write suitable code to achieve this transformation as shown below:
INPUT: OUTPUT:
OOXOO OOXOO
OOXOO OOXOO
XXXOO OOXXX
OOOOO OOOOO
XOABC CBAOX
import java.util.Scanner;
public class Question94{
public static void main(String args[]){
Scanner sc = new Scanner(System.in);
// Declaring 5x5 2D char array to store input
char original[][]= new char[5][5];
// Declaring 5x5 2D char array to store reflection
char reflection[][]= new char[5][5];
// Input 2D Array using Scanner Class
for(int line=0;line<5; line++){
String input = sc.nextLine();
char seq[] = input.toCharArray();
if(seq.length==5){
for(int i=0;i<5;i++){
original[line][i]=seq[i];
}
}
}
// Performing the reflection operation
for(int i=0; i<5;i++){
for(int j=0; j<5;j++){
reflection[i][j]=original[i][4-j];
}
}
// Output the 2D Reflection Array
for(int i=0; i<5;i++){
for(int j=0; j<5;j++){
System.out.print(reflection[i][j]);
}
System.out.println();
}
}
}
Course Name : “Programming in Java 2023”
Question : 3 Complete the code to perform a 45 degree anti clock wise rotation with respect to the center of a 5 × 5 2D Array as shown below:
INPUT:
00100
00100
11111
00100
00100
OUTPUT:
10001
01010
00100
01010
10001
import java.util.Scanner;
public class Question93{
public static void main(String args[]){
Scanner sc = new Scanner(System.in);
char arr[][]= new char[5][5];
// Input 2D Array using Scanner Class
for(int line=0;line<5; line++){
String input = sc.nextLine();
char seq[] = input.toCharArray();
if(seq.length==5){
for(int i=0;i<5;i++){
arr[line][i]=seq[i];
}
}else{
System.out.print("Wrong Input!");
System.exit(0);
}
}
// Declaring the array to store Transition
char tra[][] = new char[5][5];
String outer[]={"00","10","20","30",
"40","41","42","43",
"44","34","24","14",
"04","03","02","01"};
String inner[]={"11","21","31","32",
"33","23","13","12"};
// 45-Degree rotation
for(int i=0;i<5;i++){
for(int j=0;j<5;j++){
// Transform outer portion
for(int k=0; k
Course Name : “Programming in Java 2023”
Question : 4 Complete the code to develop an ADVANCED CALCULATOR that emulates all the functions of the GUI Calculator as shown in the image.
Input: klgc
Output: 18.0
import java.util.Scanner;
public class Question92{
public static void main(String args[]){
Scanner sc = new Scanner(System.in);
String input = sc.nextLine();
char seq[] = input.toCharArray();
int outflag=0;
// Start the mapping process for each input character
for(int i=0; i
Course Name : “Programming in Java 2023”
Question : 5 Complete the code to develop a BASIC CALCULATOR that can perform operations like Addition, Subtraction, Multiplication and Division.
Input: 5+6
Output: 5+6 = 11
import java.util.Scanner;
public class Question91{
public static void main(String args[]){
Scanner sc = new Scanner(System.in);
String input = sc.nextLine();
int i=0,j=0;
double output=0;
// Split the input string into character array
char seq[] = input.toCharArray();
/*
Use some method to separate the two operands
and then perform the required operation.
*/
for(int a=0; a