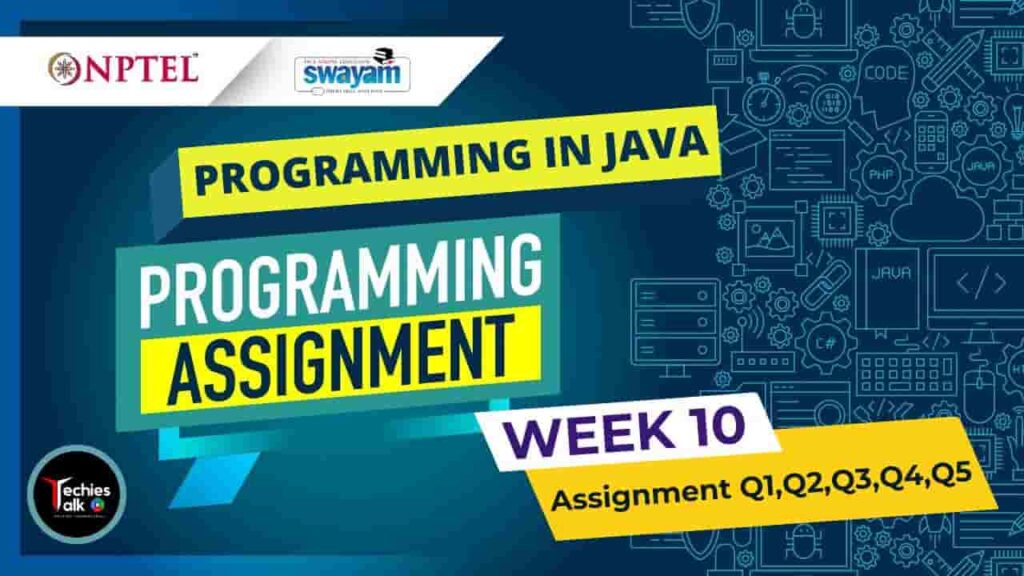
NPTEL Programming in Java Week 10 All Programming Assignment Solutions – January 2023 | Swayam. With the growth of Information and Communication Technology, there is a need to develop large and complex software.
Further, those software should be platform independent, Internet enabled, easy to modify, secure, and robust. To meet this requirement object-oriented paradigm has been developed and based on this paradigm the Java programming language emerges as the best programming environment.
Now, Java programming language is being used for mobile programming, Internet programming, and many other applications compatible to distributed systems.
This course aims to cover the essential topics of Java programming so that the participants can improve their skills to cope with the current demand of IT industries and solve many problems in their own filed of studies.
COURSE LAYOUT
- Week 1 : Overview of Object-Oriented Programming and Java
- Week 2 : Java Programming Elements
- Week 3 : Input-Output Handling in Java
- Week 4 : Encapsulation
- Week 5 : Inheritance
- Week 6 : Exception Handling
- Week 7 : Multithreaded Programming
- Week 8 : Java Applets and Servlets
- Week 9 : Java Swing and Abstract Windowing Toolkit (AWT)
- Week 10 : Networking with Java
- Week 11: Java Object Database Connectivity (ODBC)
- Week 12: Interface and Packages for Software Development
Course Name : “Programming in Java 2023”
Question : 1 The following code needs some package to work properly. Write appropriate code to import the required package(s) in order to make the program compile and execute successfully.
// Import required packages
import java.sql.*;
import java.lang.*;
public class Question101 {
public static void main(String args[]) {
try {
Connection conn = null;
Statement stmt = null;
String DB_URL = "jdbc:sqlite:/tempfs/db";
System.setProperty("org.sqlite.tmpdir", "/tempfs");
// JDBC Codes in the hidden section
// Open a connection
conn = DriverManager.getConnection(DB_URL);
System.out.print(conn.isValid(1));
conn.close();
// JDBC Codes in the visible section
}
catch(Exception e){ System.out.println(e);}
}
}
Question : 2 Write the JDBC codes needed to create a Connection interface using the DriverManager class and the variable DB_URL. Check whether the connection is successful using ‘isAlive(timeout)‘ method to generate the output, which is either ‘true’ or ‘false’.
import java.sql.*;
import java.lang.*;
import java.util.Scanner;
public class Question102 {
public static void main(String args[]) {
try {
Connection conn = null;
Statement stmt = null;
String DB_URL = "jdbc:sqlite:/tempfs/db";
System.setProperty("org.sqlite.tmpdir", "/tempfs");
// Open a connection and check connection status
conn = DriverManager.getConnection(DB_URL);
System.out.print(conn.isValid(1));
// Private test case
Scanner sc = new Scanner(System.in);
int s=sc.nextInt();
if(s==7)
{
conn.close();
System.out.print(conn.isValid(1));
}
}
catch(Exception e){ System.out.println(e);}
}
}
Question : 3 Due to some mistakes in the below code, the code is not compiled/executable. Modify and debug the JDBC code to make it execute successfully.
import java.sql.*; // All sql classes are imported
import java.lang.*; // Semicolon is added
import java.util.Scanner;
public class Question103 {
public static void main(String args[]) {
try {
Connection conn = null;
Statement stmt = null;
String DB_URL = "jdbc:sqlite:/tempfs/db";
System.setProperty("org.sqlite.tmpdir", "/tempfs");
// Connection object is created
conn = DriverManager.getConnection(DB_URL);
conn.close();
System.out.print(conn.isClosed());
}
catch(Exception e){ System.out.println(e);}
}
}
Question : 4 Complete the code segment to create a new table named ‘PLAYERS’ in SQL database using the following information.
import java.sql.*;
import java.lang.*;
public class CreateTable {
public static void main(String args[]) {
try{
Connection conn = null;
Statement stmt = null;
String DB_URL = "jdbc:sqlite:/tempfs/db";
System.setProperty("org.sqlite.tmpdir", "/tempfs");
// Open a connection
conn = DriverManager.getConnection(DB_URL);
stmt = conn.createStatement();
// The statement containing SQL command to create table "players"
String CREATE_TABLE_SQL="CREATE TABLE players (UID INT, First_Name VARCHAR(45), Last_Name VARCHAR(45), Age INT);";
// Execute the statement containing SQL command
stmt.executeUpdate(CREATE_TABLE_SQL);
ResultSet rs = stmt.executeQuery("SELECT * FROM players;");
ResultSetMetaData rsmd = rs.getMetaData();
System.out.println("No. of columns : " + rsmd.getColumnCount());
System.out.println("Column 1 Name: " + rsmd.getColumnName(1));
System.out.println("Column 1 Type : " + rsmd.getColumnTypeName(1));
System.out.println("Column 2 Name: " + rsmd.getColumnName(2));
System.out.println("Column 2 Type : " + rsmd.getColumnTypeName(2));
System.out.println("Column 3 Name: " + rsmd.getColumnName(3));
System.out.println("Column 3 Type : " + rsmd.getColumnTypeName(3));
System.out.println("Column 4 Name: " + rsmd.getColumnName(4));
System.out.println("Column 5 Type : " + rsmd.getColumnTypeName(4));
stmt.close();
conn.close();
}
catch(Exception e){ System.out.println(e);}
}
}
Question : 5 Complete the code segment to rename an already created table named ‘PLAYERS’ into ‘SPORTS’.
import java.sql.*;
import java.lang.*;
public class RenameTable {
public static void main(String args[]) {
try {
Connection conn = null;
Statement stmt = null;
String DB_URL = "jdbc:sqlite:/tempfs/db";
System.setProperty("org.sqlite.tmpdir", "/tempfs");
// Open a connection
conn = DriverManager.getConnection(DB_URL);
stmt = conn.createStatement();
// The statement containing SQL command to create table "players"
String CREATE_TABLE_SQL="CREATE TABLE players (UID INT, First_Name VARCHAR(45), Last_Name VARCHAR(45), Age INT);";
// Execute the statement containing SQL command
stmt.executeUpdate(CREATE_TABLE_SQL);
// Write the SQL command to rename a table
String alter="ALTER TABLE players RENAME TO sports;";
// Execute the SQL command
stmt.executeUpdate(alter);
ResultSet rs = stmt.executeQuery("SELECT * FROM sports;");
ResultSetMetaData rsmd = rs.getMetaData();
System.out.println("No. of columns : " + rsmd.getColumnCount());
System.out.println("Column 1 Name: " + rsmd.getColumnName(1));
System.out.println("Column 1 Type : " + rsmd.getColumnTypeName(1));
System.out.println("Column 2 Name: " + rsmd.getColumnName(2));
System.out.println("Column 2 Type : " + rsmd.getColumnTypeName(2));
System.out.println("Column 3 Name: " + rsmd.getColumnName(3));
System.out.println("Column 3 Type : " + rsmd.getColumnTypeName(3));
System.out.println("Column 4 Name: " + rsmd.getColumnName(4));
System.out.println("Column 5 Type : " + rsmd.getColumnTypeName(4));
stmt.close();
conn.close();
}
catch(Exception e){ System.out.println(e);}
}
}