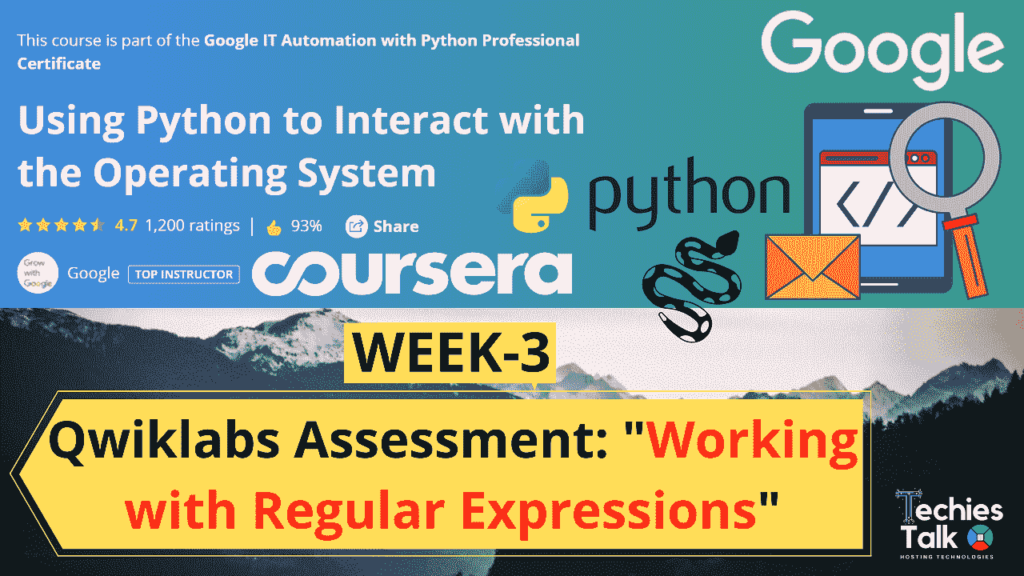
Using Python to Interact with the Operating System WEEK 3 Qwiklabs Assessment Coursera by Google. Welcome to Using Python to Interact with the Operating System. You’re joining thousands of learners currently enrolled in the course. This course is a part of Google IT Automation with Python Professional Certificate. Qwiklabs Assessment : “Working with Regular Expressions”
I’m excited to have you on my channel and look forward to your contributions to the learning community. By the end of this course:
- you’ll be able to manipulate files and processes on your computer’s operating system. You’ll also have learned about regular expressions a very powerful tool for processing text files
- you’ll get practice using the Linux command line on a virtual machine.
And, this might feel like a stretch right now, but you’ll also write a program that processes a bunch of errors in an actual log file and then generates a summary file. That’s a super useful skill for IT Specialists to know.
-:Skills you will learn:-
- Setting up your Development Environment
- Regular Expression (REGEX)
- Testing in Python
- Automating System Administration Tasks with Python
- Bash Scripting
~Course Link: https://www.coursera.org/learn/python-operating-system
Qwiklabs Assessment : “Working with Regular Expressions”
Codes:
#!/usr/bin/env python3
import re
import csv
def contains_domain(address, domain):
"""Returns True if the email address contains the given,domain,in the domain position, false if not."""
domain = r'[\w\.-]+@'+domain+'$'
if re.match(domain,address):
return True
return False
def replace_domain(address, old_domain, new_domain):
"""Replaces the old domain with the new domain in the received address."""
old_domain_pattern = r'' + old_domain + '$'
address = re.sub(old_domain_pattern, new_domain, address)
return address
def main():
"""Processes the list of emails, replacing any instances of the old domain with the new domain."""
old_domain, new_domain = 'abc.edu', 'xyz.edu'
csv_file_location = ''
report_file = '' + '/updated_user_emails.csv'
user_email_list = []
old_domain_email_list = []
new_domain_email_list = []
with open(csv_file_location, 'r') as f:
user_data_list = list(csv.reader(f))
user_email_list = [data[1].strip() for data in user_data_list[1:]]
for email_address in user_email_list:
if contains_domain(email_address, old_domain):
old_domain_email_list.append(email_address)
replaced_email = replace_domain(email_address,old_domain,new_domain)
new_domain_email_list.append(replaced_email)
email_key = ' ' + 'Email Address'
email_index = user_data_list[0].index(email_key)
for user in user_data_list[1:]:
for old_domain, new_domain in zip(old_domain_email_list, new_domain_email_list):
if user[email_index] == ' ' + old_domain:
user[email_index] = ' ' + new_domain
f.close()
with open(report_file, 'w+') as output_file:
writer = csv.writer(output_file)
writer.writerows(user_data_list)
output_file.close()
main()