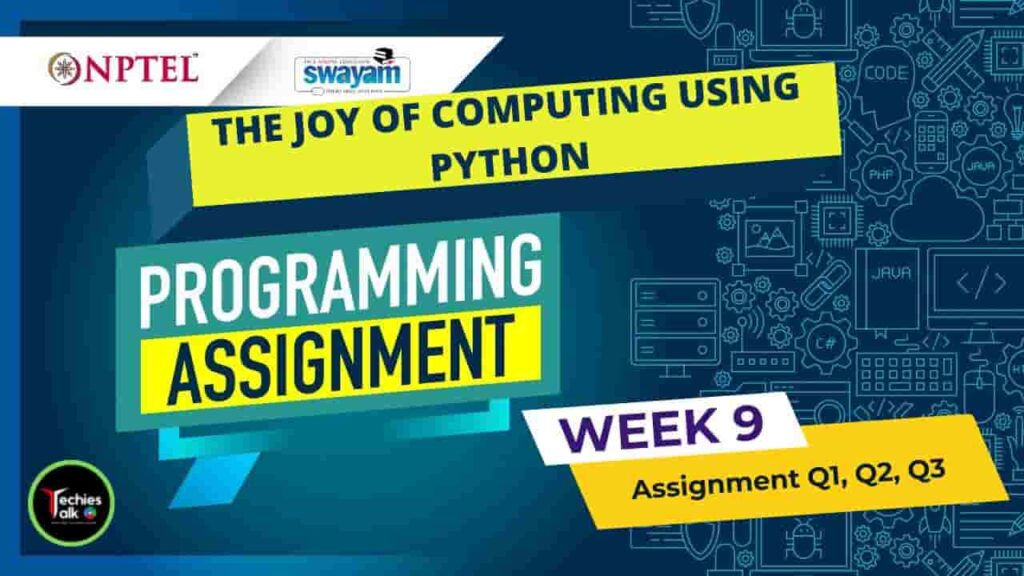
NPTEL Course a fun filled whirlwind tour of 30 hrs, covering everything you need to know to fall in love with the most sought after skill of the 21st century. The course brings programming to your desk with anecdotes, analogies and illustrious examples. Turning abstractions to insights and engineering to art, the course focuses primarily to inspire the learner’s mind to think logically and arrive at a solution programmatically. As part of the course, you will be learning how to practice and culture the art of programming with Python as a language. At the end of the course, we introduce some of the current advances in computing to motivate the enthusiastic learner to pursue further directions. The Joy of Computing using python is a fun filled course offered by NPTEL.
The Joy Of Computing Using Python Week 9 Assignment 2023.
INTENDED AUDIENCE : Any interested audience
PREREQUISITES : 10th standard/high school
INDUSTRY SUPPORT : Every software company is aware of the potential of a first course in computer science. Especially of a first course in computing, done right.
Course Layout
- Motivation for Computing
- Welcome to Programming!!
- Variables and Expressions : Design your own calculator
- Loops and Conditionals : Hopscotch once again
- Lists, Tuples and Conditionals : Lets go on a trip
- Abstraction Everywhere : Apps in your phone
- Counting Candies : Crowd to the rescue
- Birthday Paradox : Find your twin
- Google Translate : Speak in any Language
- Currency Converter : Count your foreign trip expenses
- Monte Hall : 3 doors and a twist
- Sorting : Arrange the books
- Searching : Find in seconds
- Substitution Cipher : What’s the secret !!
- Sentiment Analysis : Analyse your Facebook data
- 20 questions game : I can read your mind
- Permutations : Jumbled Words
- Spot the similarities : Dobble game
- Count the words : Hundreds, Thousands or Millions.
- Rock, Paper and Scissor : Cheating not allowed !!
- Lie detector : No lies, only TRUTH
- Calculation of the Area : Don’t measure.
- Six degrees of separation : Meet your favourites
- Image Processing : Fun with images
- Tic tac toe : Let’s play
- Snakes and Ladders : Down the memory lane.
- Recursion : Tower of Hanoi
- Page Rank : How Google Works !!
Programming Assignment 1
Question 1 : Given two strings s1 and s2, write a function subStr that accepts two strings s1 and s2 and will return True if a s2 is a substring of s1 otherwise return False. A substring is a is a contiguous sequence of characters within a string.
Input:
bananamania
nana
Output:
True
def subStr(s1, s2):
M = len(s1)
N = len(s2)
for i in range(M - N + 1):
flag = True
for j in range(N):
if (s1[i + j] != s2[j]):
flag = False
break
if flag :
return True
return False
if __name__ == "__main__":
s1 = input()
s2 = input()
print(subStr(s1, s2),end="")
Programming Assignment 2
Question 2 : Given two dictionaries d1 and d2, write a function mergeDic that accepts two dictionaries d1 and d2 and return a new dictionary by merging d1 and d2.
Note: Contents of d1 should be appear before contents of d2 in the new dictionary and in same order. In case of duplicate value retain the value present in d1.
Input:
{1: 1, 2: 2}
output:
{1: 1, 2: 2, 3: 3, 4: 4}
def mergeDic(d1, d2):
dic = {}
for key,value in d1.items():
dic[key] = value
for key,value in d2.items():
if key not in dic.keys():
dic[key] = value
return dic
key = list(map(int, input().split()))
val = list(map(int, input().split()))
d1 = {}
for i in range(len(key)):
d1[key[i]] = val[i]
d2 = {}
key = list(map(int, input().split()))
val = list(map(int, input().split()))
for i in range(len(key)):
d2[key[i]] = val[i]
print(mergeDic(d1, d2))
Programming Assignment 3
Question 3 : Given an integer N, print all the indexes of numbers in that integer from left to right.
Input:
122345
Output:
1 0
2 1 2
3 3
4 4
5 5
n = str(input())
dic = {}
for i in range(len(n)):
if n[i] not in dic.keys():
dic[n[i]] = [i]
else:
dic[n[i]].append(i)
for key, value in dic.items():
print(key, end= ' ')
for i in value:
print(i, end= ' ')
print()