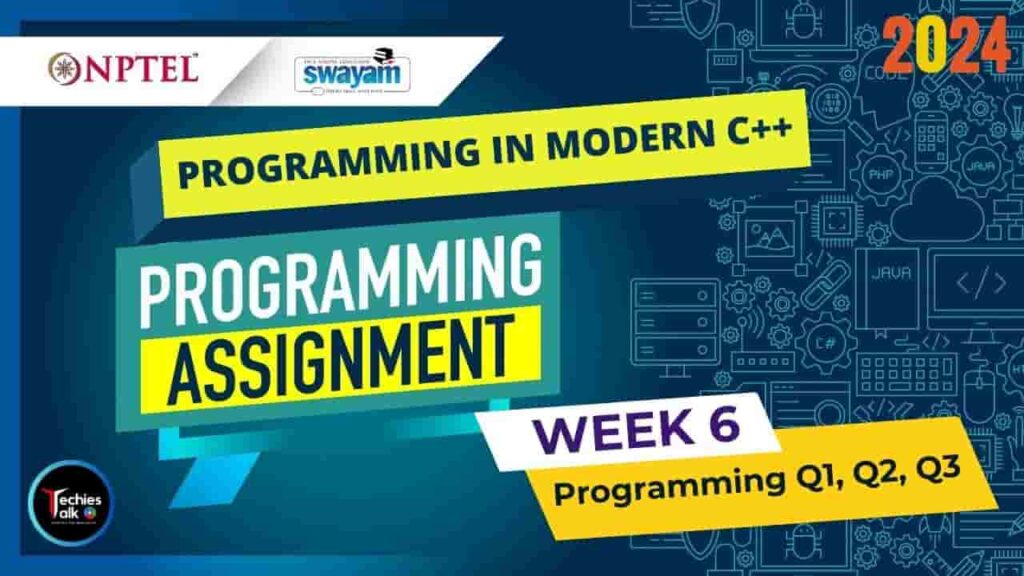
There has been a continual debate on which programming language/s to learn, to use. As the latest TIOBE Programming Community Index for August 2021 indicates – C (13%), Python (12%), C++ (7%), Java (10%), and C#(5%) together control nearly half the programming activities worldwide. Further, C Programming Language Family (C, C++, C#, Objective C etc.) dominate more than 25% of activities. Hence, learning C++ is important as one learns about the entire family, about Object-Oriented Programming and gets a solid foundation to also migrate to Java and Python as needed. C++ is the mother of most general purpose of languages. It is multi-paradigm encompassing procedural, object-oriented, generic, and even functional programming. C++ has primarily been the systems language till C++03 which punches efficiency of the code with the efficacy of OOP. Then, why should I learn it if my primary focus is on applications? This is where the recent updates of C++, namely, C++11 and several later offer excellent depths and flexibility for C++ that no language can match. These extensions attempt to alleviate some of the long-standing shortcomings for C++ including porous resource management, error-prone pointer handling, expression semantics, and better readability. The present course builds up on the knowledge of C programming and basic data structure (array, list, stack, queue etc.) to create a strong familiarity with C++98 / C++03. Besides the constructs, syntax and semantics of C++ (over C), we also focus on various idioms of C++ and attempt to go to depth with every C++ feature justifying and illustrating them with several examples and assignment problems. On the way, we illustrate various OOP concepts. The course also covers important advances in C++11 and later released features..
Programming In Modern C++ Week6 Programming Assignment 2024
INTENDED AUDIENCE : Any interested audience
PREREQUISITES : 10th standard/high school
INDUSTRY SUPPORT : Programming in C++ is so fundamental that all companies dealing with systems as well as application development (including web, IoT, embedded systems) have a need for the same. These include – Microsoft, Samsung, Xerox, Yahoo, Oracle, Google, IBM, TCS, Infosys, Amazon, Flipkart, etc. This course would help industry developers to be up-to-date with the advances in C++ so that they can remain at the state-of-the-art.
Course Layout
Programming In Modern C++ 2024
Programming Assignment Q1 :
Complete the program with the following instructions.
• Fill in the blank at LINE-1 with appropriate keyword,
• Fill in the blanks at LINE-2 to declare fun() as a pure virtual function,
• fill in the blank at LINE-3 with approriate object instantiation,
The program must satisfy the given test cases
#include
using namespace std;
class Base{
protected:
int s;
public:
Base(int i=0) : s(i){}
virtual ~Base(){ } //LINE-1
virtual int fun() = 0; //LINE-2
};
class Derived : public Base{
int x;
public:
Derived(int i, int j) : Base(i), x(j) {}
~Derived();
int fun(){
return s*x;
}
};
class Wrapper{
public:
void fun(int a, int b){
Base *t = new Derived(a, b); //LINE-3
int i = t->fun();
cout << i << " ";
delete t;
}
};
Derived::~Derived(){ cout << int(s/x) << " "; }
int main(){
int i, j;
cin >> i >> j;
Wrapper w;
w.fun(i,j);
return 0;
}
Programming In Modern C++ 2024
Programming Assignment Q2 :
Consider the following program with the following instructions.
Fill in the blank at LINE-1 and LINE-2 with appropriate return statements
The program must satisfy the sample input and output.
#include
using namespace std;
const double ir = 7;
const double bonus = 4;
class FD{
protected:
double principal;
public:
FD(double _p = 0.0) : principal(_p){}
double getInterest(){ return principal * ir / 100; }
double getExtraAmt(){ return principal * bonus / 100 ; }
virtual double getMaturity() = 0;
};
class Customer : public FD{
public:
Customer(double _amt) : FD(_amt){}
double getMaturity();
};
class Employee : public FD{
public:
Employee(double _amt) : FD(_amt){}
double getMaturity();
};
double Customer::getMaturity(){
return principal + getInterest(); //LINE-1
}
double Employee::getMaturity(){
return principal + getInterest() + getExtraAmt(); //LINE-2
}
int main(){
double d;
cin >> d;
FD *ep[2] = {new Customer(d), new Employee(d)};
cout << ep[0]->getMaturity() << " ";
cout << ep[1]->getMaturity();
return 0;
}
Programming In Modern C++ 2024
Programming Assignment Q3 :
Consider the following program. Fill in the blanks as per the instructions given below:
• Fill in the blank at LINE-1 with an appropriate destructor declaration.
• Fill in the blank at LINE-2 with an appropriate constructor statement.
such that it will satisfy the given test cases.
#include
using namespace std;
class A{
public:
A(){ cout << "11 "; }
A(int n){ cout << n + 2 << " "; }
virtual ~A(); //LINE-1
};
class B : public A{
public:
B(int n) : A(n) //LINE-2
{
cout << n + 5 << " ";
}
B(){ cout << "21 "; }
virtual ~B(){ cout << "22 "; }
};
A::~A(){ cout << "12 "; }
int main(){
int i;
cin >> i;
A *pt = new B(i);
delete pt;
return 0;
}