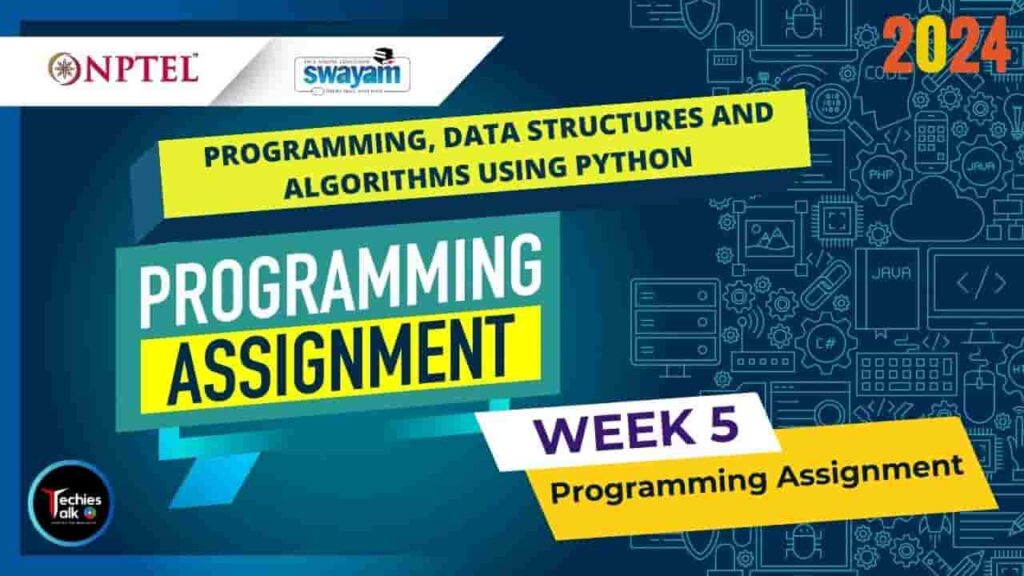
NPTEL Course is an introduction to programming and problem solving in Python. It does not assume any prior knowledge of programming. Using some motivating examples, the course quickly builds up basic concepts such as conditionals, loops, functions, lists, strings and tuples. It goes on to cover searching and sorting algorithms, dynamic programming and backtracking, as well as topics such as exception handling and using files. As far as data structures are concerned, the course covers Python dictionaries as well as classes and objects for defining user defined datatypes such as linked lists and binary search trees.
Programming, Data Structures And Algorithms Using Python Week5 Assignment Jan 2024
INTENDED AUDIENCE : Students in any branch of mathematics/science/engineering, 1st year
PREREQUISITES : School level mathematics
INDUSTRY SUPPORT : This course should be of value to any company requiring programming skills.
Course Layout
Week 1:
Informal introduction to programming, algorithms and data structures via GCD
Downloading and installing Python
GCD in Python: variables, operations, control flow – assignments, conditionals, loops, functions
Week 2:
Python: types, expressions, strings, lists, tuples
Python memory model: names, mutable and immutable values
List operations: slices etc
Binary search
Inductive function definitions: numerical and structural induction
Elementary inductive sorting: selection and insertion sort
In-place sorting
Week 3:
Basic algorithmic analysis: input size, asymptotic complexity, O() notation
Arrays vs lists
Merge sort
Quicksort
Stable sorting
Week 4:
Dictionaries
More on Python functions: optional arguments, default values
Passing functions as arguments
Higher order functions on lists: map, lter, list comprehension
Week 5:
Exception handling
Basic input/output
Handling files
String processing
Week 6:
Backtracking: N Queens, recording all solutions
Scope in Python: local, global, non-local names
Nested functions
Data structures: stack, queue
Heaps
Week 7:
Abstract data-types
Classes and objects in Python
“Linked” lists: find, insert, delete
Binary search trees: find, insert, delete
Height-balanced binary search trees
Week 8:
Efficient evaluation of recursive definitions: memorization
Dynamic programming: examples
Other programming languages: C and manual memory management
Other programming paradigms: functional programming
Programming Assignment 1
For this assignment, you have to write a complete Python program. Paste your code in the window below.
- You may define additional auxiliary functions as needed.
- There are some public test cases and some (hidden) private test cases.
- “Compile and run” will evaluate your submission against the public test cases
- “Submit” will evaluate your submission against the hidden private test cases. There are 6 private test cases, with equal weightage. You will get feedback about which private test cases pass or fail, though you cannot see the actual test cases.
- Ignore warnings about “Presentation errors”.
Here are some basic facts about tennis scoring: A tennis match is made up of sets. A set is made up of games.
To win a set, a player has to win 6 games with a difference of 2 games. At 6-6, there is often a special tie-breaker. In some cases, players go on playing till one of them wins the set with a difference of two games.
Tennis matches can be either 3 sets or 5 sets. The player who wins a majority of sets wins the match (i.e., 2 out 3 sets or 3 out of 5 sets) The score of a match lists out the games in each set, with the overall winner’s score reported first for each set. Thus, if the score is 6-3, 5-7, 7-6 it means that the first player won the first set by 6 games to 3, lost the second one 5 games to 7 and won the third one 7 games to 6 (and hence won the overall match as well by 2 sets to 1).
You will read input from the keyboard (standard input) containing the results of several tennis matches. Each match’s score is recorded on a separate line with the following format:
Winner:Loser:Set-1-score,...,Set-k-score, where 2 ≤ k ≤ 5
For example, an input line of the form
Jabeur:Swiatek:3-6,6-3,6-3
indicates that Jabeur beat Swiatek 3-6, 6-3, 6-3 in a best of 3 set match.
The input is terminated by a line consisting of the string “EOF”.
You have to write a Python program that reads information about all the matches and compile the following statistics for each player:
- Number of best-of-5 set matches won
- Number of best-of-3 set matches won
- Number of sets won
- Number of games won
- Number of sets lost
- Number of games lost
You should print out to the screen (standard output) a summary in decreasing order of ranking, where the ranking is according to the criteria 1-6 in that order (compare item 1, if equal compare item 2, if equal compare item 3 etc, noting that for items 5 and 6 the comparison is reversed).
For instance, given the following data
Zverev:Alcaraz:2-6,6-7,7-6,6-3,6-1 Swiatek:Jabeur:6-4,6-4 Alcaraz:Zverev:6-3,6-3 Jabeur:Swiatek:1-6,7-5,6-2 Zverev:Alcaraz:6-0,7-6,6-3 Jabeur:Swiatek:2-6,6-2,6-0 Alcaraz:Zverev:6-3,4-6,6-3,6-4 Swiatek:Jabeur:6-1,3-6,7-5 Zverev:Alcaraz:7-6,4-6,7-6,2-6,6-2 Jabeur:Swiatek:6-4,1-6,6-3 Alcaraz:Zverev:7-5,7-5 Jabeur:Swiatek:3-6,6-3,6-3 EOF
your program should print out the following
Zverev 3 0 10 104 11 106 Alcaraz 1 2 11 106 10 104 Jabeur 0 4 9 76 8 74 Swiatek 0 2 8 74 9 76
You can assume that there are no spaces around the punctuation marks “:”, “-” and “,”. Each player’s name will be spelled consistently and no two players have the same name.
def process_matches():
stats = {}
line_input = input()
while line_input != "EOF":
winner_sets, loser_sets, win_games, lose_games = 0, 0, 0, 0
winner, loser, setscores = line_input.strip().split(':', 2)
sets = setscores.split(',')
for set_score in sets:
win_str, lose_str = map(int, set_score.split('-'))
win_games += win_str
lose_games += lose_str
if win_str > lose_str:
winner_sets += 1
else:
loser_sets += 1
for player in [winner, loser]:
if player not in stats:
stats[player] = [0, 0, 0, 0, 0, 0]
if winner_sets >= 3:
stats[winner][0] += 1
else:
stats[winner][1] += 1
stats[winner][2] += winner_sets
stats[winner][3] += win_games
stats[winner][4] -= loser_sets
stats[winner][5] -= lose_games
stats[loser][2] += loser_sets
stats[loser][3] += lose_games
stats[loser][4] -= winner_sets
stats[loser][5] -= win_games
line_input = input()
return stats
def print_summary(stats):
statlist = [(stat[0], stat[1], stat[2], stat[3], stat[4], stat[5], name)
for name in stats.keys() for stat in [stats[name]]]
statlist.sort(reverse=True)
for entr in statlist:
print(entr[6], entr[0], entr[1], entr[2], entr[3], -entr[4], -entr[5])
if __name__ == "__main__":
matches = process_matches()
print_summary(matches)