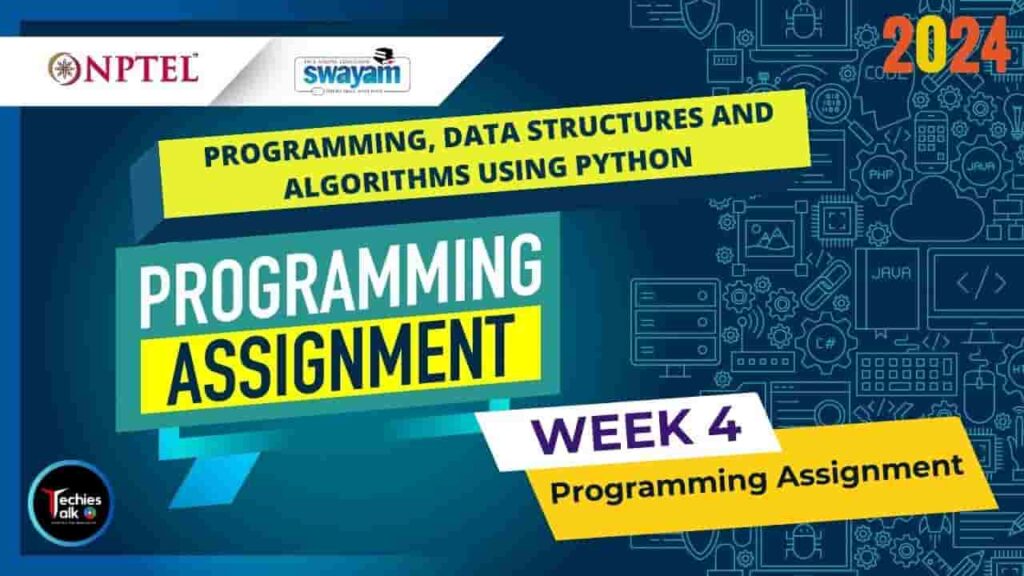
NPTEL Course is an introduction to programming and problem solving in Python. It does not assume any prior knowledge of programming. Using some motivating examples, the course quickly builds up basic concepts such as conditionals, loops, functions, lists, strings and tuples. It goes on to cover searching and sorting algorithms, dynamic programming and backtracking, as well as topics such as exception handling and using files. As far as data structures are concerned, the course covers Python dictionaries as well as classes and objects for defining user defined datatypes such as linked lists and binary search trees.
Programming, Data Structures And Algorithms Using Python Week4 Assignment Jan 2024
INTENDED AUDIENCE : Students in any branch of mathematics/science/engineering, 1st year
PREREQUISITES : School level mathematics
INDUSTRY SUPPORT : This course should be of value to any company requiring programming skills.
Course Layout
Week 1:
Informal introduction to programming, algorithms and data structures via GCD
Downloading and installing Python
GCD in Python: variables, operations, control flow – assignments, conditionals, loops, functions
Week 2:
Python: types, expressions, strings, lists, tuples
Python memory model: names, mutable and immutable values
List operations: slices etc
Binary search
Inductive function definitions: numerical and structural induction
Elementary inductive sorting: selection and insertion sort
In-place sorting
Week 3:
Basic algorithmic analysis: input size, asymptotic complexity, O() notation
Arrays vs lists
Merge sort
Quicksort
Stable sorting
Week 4:
Dictionaries
More on Python functions: optional arguments, default values
Passing functions as arguments
Higher order functions on lists: map, lter, list comprehension
Week 5:
Exception handling
Basic input/output
Handling files
String processing
Week 6:
Backtracking: N Queens, recording all solutions
Scope in Python: local, global, non-local names
Nested functions
Data structures: stack, queue
Heaps
Week 7:
Abstract data-types
Classes and objects in Python
“Linked” lists: find, insert, delete
Binary search trees: find, insert, delete
Height-balanced binary search trees
Week 8:
Efficient evaluation of recursive definitions: memorization
Dynamic programming: examples
Other programming languages: C and manual memory management
Other programming paradigms: functional programming
Programming Assignment 1
Write Python functions as specified below. Paste the text for all functions together into the submission window.
- You may define additional auxiliary functions as needed.
- In all cases you may assume that the value passed to the function is of the expected type, so your function does not have to check for malformed inputs.
- For each function, there are some public test cases and some (hidden) private test cases.
- “Compile and run” will evaluate your submission against the public test cases.
- “Submit” will evaluate your submission against the hidden private test cases and report a score on 100. There are 10 private testcases in all, each with equal weightage. You will get feedback about which private test cases pass or fail, though you cannot see the actual test cases.
- Ignore warnings about “Presentation errors”.
We represent scores of batsmen across a sequence of matches in a two level dictionary as follows:
{'match1':{'player1':57, 'player2':38}, 'match2':{'player3':9, 'player1':42}, 'match3':{'player2':41, 'player4':63, 'player3':91}
Each match is identified by a string, as is each player. The scores are all integers. The names associated with the matches are not fixed (here they are 'match1', 'match2', 'match3'), nor are the names of the players. A player need not have a score recorded in all matches.
Define a Python function orangecap(d) that reads a dictionary d of this form and identifies the player with the highest total score. Your function should return a pair (playername,topscore) where playername is a string, the name of the player with the highest score, and topscore is an integer, the total score of playername.
The input will be such that there are never any ties for highest total score.
For instance:
>>> orangecap({'match1':{'player1':57, 'player2':38}, 'match2':{'player3':9, 'player1':42}, 'match3':{'player2':41, 'player4':63, 'player3':91}}) ('player3', 100) >>> orangecap({'test1':{'Pant':84, 'Kohli':120}, 'test2':{'Pant':59, 'Gill':42}}) ('Pant', 143)
Let us consider polynomials in a single variable x with integer coefficients. For instance:
3x4 - 17x2 - 3x + 5
Each term of the polynomial can be represented as a pair of integers (coefficient,exponent). The polynomial itself is then a list of such pairs.
We have the following constraints to guarantee that each polynomial has a unique representation:
- Terms are sorted in descending order of exponent
- No term has a zero cofficient
- No two terms have the same exponent
- Exponents are always nonnegative
For example, the polynomial introduced earlier is represented as:
[(3,4),(-17,2),(-3,1),(5,0)]
The zero polynomial, 0, is represented as the empty list [], since it has no terms with nonzero coefficients.
Write Python functions for the following operations:
addpoly(p1,p2) multpoly(p1,p2)
that add and multiply two polynomials, respectively.
You may assume that the inputs to these functions follow the representation given above. Correspondingly, the outputs from these functions should also obey the same constraints.
You can write auxiliary functions to “clean up” polynomials – e.g., remove zero coefficient terms, combine like terms, sort by exponent etc. Build a library of functions that can be combined to achieve the desired format.
You may also want to convert the list representation to a dictionary representation and manipulate the dictionary representation, and then convert back.
Some examples:
>>> addpoly([(4,3),(3,0)],[(-4,3),(2,1)]) [(2, 1),(3, 0)] Explanation: (4x^3 + 3) + (-4x^3 + 2x) = 2x + 3 >>> addpoly([(2,1)],[(-2,1)]) [] Explanation: 2x + (-2x) = 0 >>> multpoly([(1,1),(-1,0)],[(1,2),(1,1),(1,0)]) [(1, 3),(-1, 0)] Explanation: (x - 1) * (x^2 + x + 1) = x^3 - 1
# Function to find the player with the highest total score
def orangecap(d):
scores = {}
for match in d:
for player in d[match]:
scores[player] = scores.get(player, 0) + d[match][player]
playername = max(scores, key=scores.get)
topscore = scores[playername]
return (playername, topscore)
# Function to add two polynomials
def addpoly(p1, p2):
d = {}
for coeff, exp in p1+p2:
if exp in d:
d[exp] += coeff
else:
d[exp] = coeff
result = [(coeff, exp) for exp, coeff in d.items() if coeff != 0]
result.sort(key=lambda x: x[1], reverse=True)
return result
# Function to multiply two polynomials
def multpoly(p1, p2):
d = {}
for coeff1, exp1 in p1:
for coeff2, exp2 in p2:
if exp1+exp2 in d:
d[exp1+exp2] += coeff1*coeff2
else:
d[exp1+exp2] = coeff1*coeff2
result = [(coeff, exp) for exp, coeff in d.items() if coeff != 0]
result.sort(key=lambda x: x[1], reverse=True)
return result