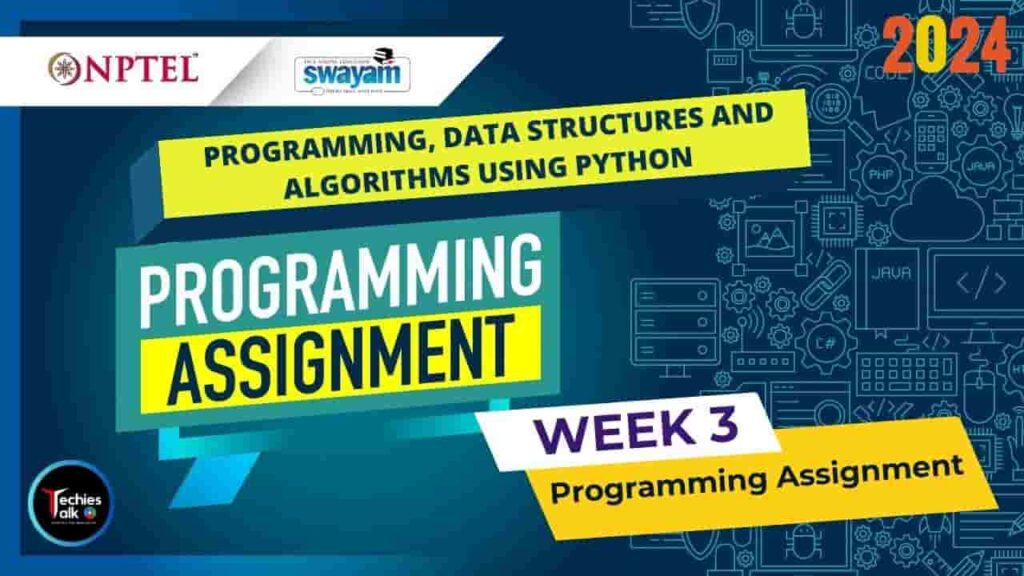
NPTEL Course is an introduction to programming and problem solving in Python. It does not assume any prior knowledge of programming. Using some motivating examples, the course quickly builds up basic concepts such as conditionals, loops, functions, lists, strings and tuples. It goes on to cover searching and sorting algorithms, dynamic programming and backtracking, as well as topics such as exception handling and using files. As far as data structures are concerned, the course covers Python dictionaries as well as classes and objects for defining user defined datatypes such as linked lists and binary search trees.
Programming, Data Structures And Algorithms Using Python Week3 Assignment Jan 2024
INTENDED AUDIENCE : Students in any branch of mathematics/science/engineering, 1st year
PREREQUISITES : School level mathematics
INDUSTRY SUPPORT : This course should be of value to any company requiring programming skills.
Course Layout
Week 1:
Informal introduction to programming, algorithms and data structures via GCD
Downloading and installing Python
GCD in Python: variables, operations, control flow – assignments, conditionals, loops, functions
Week 2:
Python: types, expressions, strings, lists, tuples
Python memory model: names, mutable and immutable values
List operations: slices etc
Binary search
Inductive function definitions: numerical and structural induction
Elementary inductive sorting: selection and insertion sort
In-place sorting
Week 3:
Basic algorithmic analysis: input size, asymptotic complexity, O() notation
Arrays vs lists
Merge sort
Quicksort
Stable sorting
Week 4:
Dictionaries
More on Python functions: optional arguments, default values
Passing functions as arguments
Higher order functions on lists: map, lter, list comprehension
Week 5:
Exception handling
Basic input/output
Handling files
String processing
Week 6:
Backtracking: N Queens, recording all solutions
Scope in Python: local, global, non-local names
Nested functions
Data structures: stack, queue
Heaps
Week 7:
Abstract data-types
Classes and objects in Python
“Linked” lists: find, insert, delete
Binary search trees: find, insert, delete
Height-balanced binary search trees
Week 8:
Efficient evaluation of recursive definitions: memorization
Dynamic programming: examples
Other programming languages: C and manual memory management
Other programming paradigms: functional programming
Programming Assignment 1
Write three Python functions as specified below. Paste the text for all three functions together into the submission window. Your function will be called automatically with various inputs and should return values as specified. Do not write commands to read any input or print any output.
- You may define additional auxiliary functions as needed.
- In all cases you may assume that the value passed to the function is of the expected type, so your function does not have to check for malformed inputs.
- For each function, there are normally some public test cases and some (hidden) private test cases.
- “Compile and run” will evaluate your submission against the public test cases.
- “Submit” will evaluate your submission against the hidden private test cases. There are 10 private test cases, with equal weightage. You will get feedback about which private test cases pass or fail, though you cannot see the actual test cases.
- Ignore warnings about “Presentation errors”.
Define a Python function remdup(l) that takes a nonempty list of integers l and removes all duplicates in l, keeping only the first occurrence of each number. For instance:
>>> remdup([3,1,3,5]) [3, 1, 5] >>> remdup([7,3,-1,-5]) [7, 3, -1, -5] >>> remdup([3,5,7,5,3,7,10]) [3, 5, 7, 10]
Write a Python function sumsquare(l) that takes a nonempty list of integers and returns a list [odd,even], where odd is the sum of squares all the odd numbers in l and even is the sum of squares of all the even numbers in l.
Here are some examples to show how your function should work.
>>> sumsquare([1,3,5]) [35, 0] >>> sumsquare([2,4,6]) [0, 56] >>> sumsquare([-1,-2,3,7]) [59, 4]
A two dimensional matrix can be represented in Python row-wise, as a list of lists: each inner list represents one row of the matrix. For instance, the matrix
1 2 3 4 5 6 7 8
would be represented as [[1, 2, 3, 4], [5, 6, 7, 8]].
The transpose of a matrix converts each row into a column. The transpose of the matrix above is:
1 5 2 6 3 7 4 8
which would be represented as [[1, 5], [2, 6], [3, 7], [4, 8]].
Write a Python function transpose(m) that takes as input a two dimensional matrix m and returns the transpose of m. The argument m should remain undisturbed by the function.
Here are some examples to show how your function should work. You may assume that the input to the function is always a non-empty matrix.
>>> transpose([[1,2,3],[4,5,6]]) [[1, 4], [2, 5], [3, 6]] >>> transpose([[1],[2],[3]]) [[1, 2, 3]] >>> transpose([[3]]) [[3]]
# Function to remove duplicates from a list
def remdup(l):
return list(dict.fromkeys(l))
# Function to calculate sum of squares of odd and even numbers
def sumsquare(l):
odd = sum(i**2 for i in l if i % 2 != 0)
even = sum(i**2 for i in l if i % 2 == 0)
return [odd, even]
# Function to transpose a matrix
def transpose(m):
return [list(i) for i in zip(*m)]