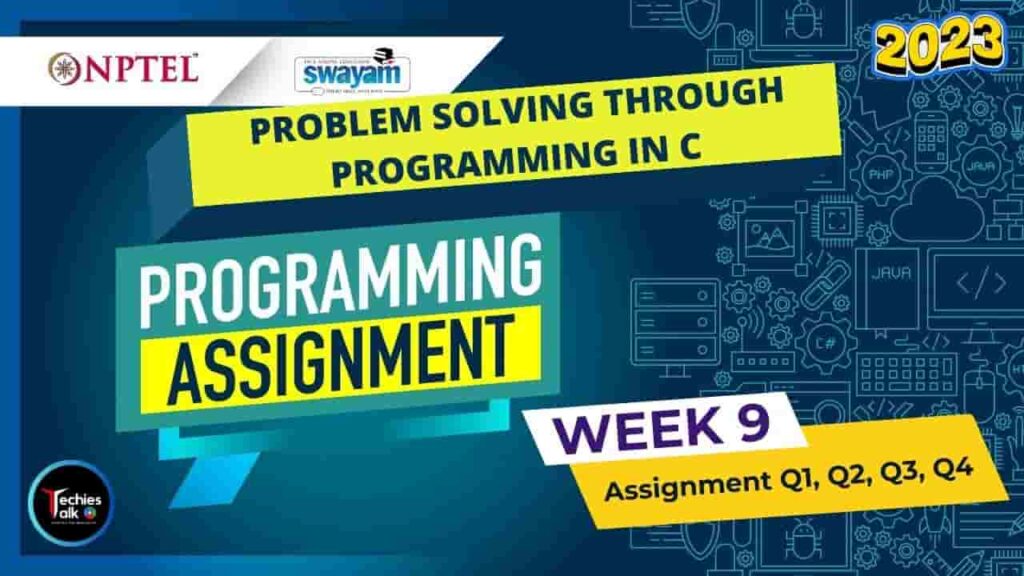
NPTEL Problem Solving Through Programming In C Week9 All Programming Assignment Solutions | Swayam July 2023. With the growth of Information and Communication Technology, there is a need to develop large and complex software.
ABOUT THE COURSE:
- Formulate simple algorithms for arithmetic and logical problems
- Translate the algorithms to programs (in C language)
- Test and execute the programs and correct syntax and logical errors
- Implement conditional branching, iteration and recursion
- Decompose a problem into functions and synthesize a complete program using divide and conquer approach
- Use arrays, pointers and structures to formulate algorithms and programs
- Apply programming to solve matrix addition and multiplication problems and searching and sorting problems
- Apply programming to solve simple numerical method problems, namely rot finding of function, differentiation of function and simple integration
COURSE LAYOUT
- Week 1 : Introduction to Problem Solving through programs, Flowcharts/Pseudo codes, the compilation process, Syntax and Semantic errors, Variables and Data Types
- Week 2 : Arithmetic expressions, Relational Operations, Logical expressions; Introduction to Conditional Branching
- Week 3 : Conditional Branching and Iterative Loops
- Week 4 : Arranging things : Arrays
- Week 5 : 2-D arrays, Character Arrays and Strings
- Week 6 : Basic Algorithms including Numerical Algorithms
- Week 7 : Functions and Parameter Passing by Value
- Week 8 : Passing Arrays to Functions, Call by Reference
- Week 9 : Recursion
- Week 10 : Structures and Pointers
- Week 11 : Self-Referential Structures and Introduction to Lists
- Week 12 : Advanced Topics
Once again, thanks for your interest in our online courses and certification. Happy learning!
Problem Solving through Programming In C July 2023
Question : 1 Write a program to print all the locations at which a particular element (taken as input) is found in a list and also print the total number of times it occurs in the list. The location starts from 1.
For Example: If there are 4 elements in the array i.e, 5 6 5 7
If the element to search is 5 then the output will be
5 is present at location 1
5 is present at location 3
5 is present 2 times in the array
#include
int main()
{
int array[100], search, n, count = 0;
scanf("%d", &n);
int c;
for (c = 0; c < n; c++)
scanf("%d", &array[c]);
scanf("%d", &search);
for (c = 0; c < n; c++)
{
if (array[c] == search)
{
printf("%d is present at location %d.\n", search, c+1);
count++;
}
}
if (count == 0)
printf("%d is not present in the array.", search);
else
printf("%d is present %d times in the array.", search, count);
return 0;
}
Problem Solving through Programming In C July 2023
Question : 2 Write a C program to search a given element from a 1D array and display the position at which it is found by using linear search function. The index location starts from 1.
#include
int linear_search(int[], int, int);
int main()
{
int array[100], search, c, n, position;
scanf("%d", &n);
for (c = 0; c < n; c++)
scanf("%d", &array[c]);
scanf("%d", &search);
position = linear_search(array, n, search);
if(position == -1)
printf("%d is not present in the array.", search);
else
printf("%d is present at location %d.", search, position+1);
return 0;
}
int linear_search(int a[], int n, int find)
{
int c;
for(c = 0; c
Problem Solving through Programming In C July 2023
Question : 3 Write a C program to search a given number from a sorted 1D array and display the position at which it is found using binary search algorithm. The index location starts from 1.
#include
int main()
{
int c, n, search,
array[100];
scanf("%d",&n); //number of elements in the array
for (c = 0; c < n; c++)
scanf("%d",&array[c]);
scanf("%d", &search);
int first, last, middle;
first = 0;
last = n - 1;
middle = (first+last)/2;
while(first <= last)
{
if(array[middle] < search)
first = middle + 1;
else if(array[middle] == search)
{
printf("%d found at location %d.", search, middle+1);
break;
}
else
last = middle - 1;
middle = (first + last)/2;
}
if(first > last)
printf("Not found! %d isn't present in the list.", search);
return 0;
}
Problem Solving through Programming In C July 2023
Question : 4 Write a C program to reverse an array by swapping the elements and without using any new array.
#include
int main()
{
int array[100], n, c;
scanf("%d", &n); // n is number of elements in the array.
for (c = 0; c < n; c++) {
scanf("%d", &array[c]);
}
int temp, end;
end = n - 1;
for(c = 0; c < n/2; c++)
{
temp = array[c];
array[c] = array[end];
array[end] = temp;
end--;
}
printf("Reversed array elements are:\n");
for (c = 0; c < n; c++) {
printf("%d\n", array[c]);
}
return 0;
}