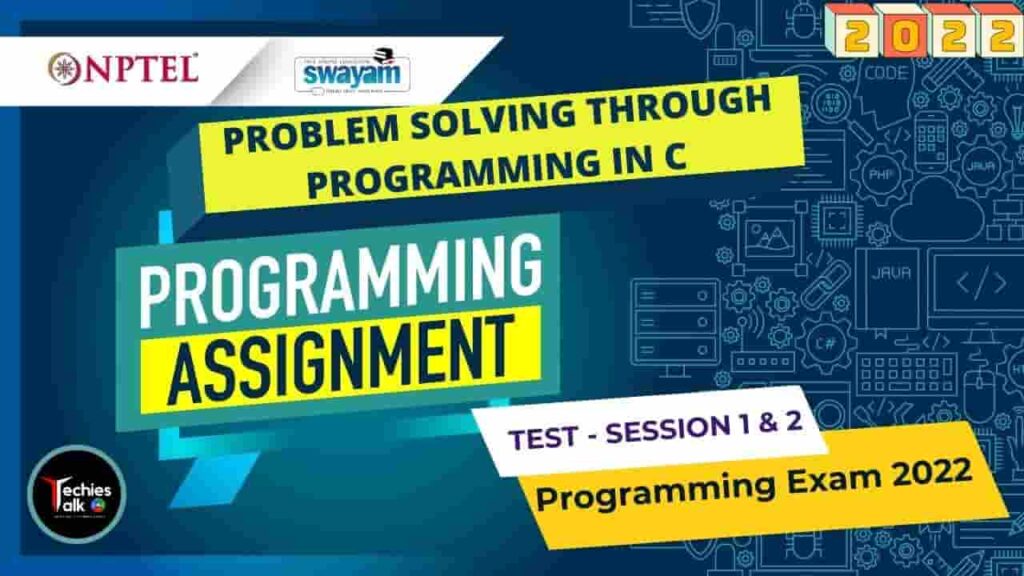
NPTEL Problem solving through Programming In C Programming Exam Unprotected Oct 2022| Swayam 2022. With the growth of Information and Communication Technology, there is a need to develop large and complex software.
ABOUT THE COURSE:
- Formulate simple algorithms for arithmetic and logical problems
- Translate the algorithms to programs (in C language)
- Test and execute the programs and correct syntax and logical errors
- Implement conditional branching, iteration and recursion
- Decompose a problem into functions and synthesize a complete program using divide and conquer approach
- Use arrays, pointers and structures to formulate algorithms and programs
- Apply programming to solve matrix addition and multiplication problems and searching and sorting problems
- Apply programming to solve simple numerical method problems, namely rot finding of function, differentiation of function and simple integration
COURSE LAYOUT
- Week 1 : Introduction to Problem Solving through programs, Flowcharts/Pseudo codes, the compilation process, Syntax and Semantic errors, Variables and Data Types
- Week 2 : Arithmetic expressions, Relational Operations, Logical expressions; Introduction to Conditional Branching
- Week 3 : Conditional Branching and Iterative Loops
- Week 4 : Arranging things : Arrays
- Week 5 : 2-D arrays, Character Arrays and Strings
- Week 6 : Basic Algorithms including Numerical Algorithms
- Week 7 : Functions and Parameter Passing by Value
- Week 8 : Passing Arrays to Functions, Call by Reference
- Week 9 : Recursion
- Week 10 : Structures and Pointers
- Week 11 : Self-Referential Structures and Introduction to Lists
- Week 12 : Advanced Topics
Once again, thanks for your interest in our online courses and certification. Happy learning!
Programming Exam Unprotected Oct 2022
Programming Exam Oct 2022 (First Session): (10AM - 11AM)
Course Name : “Problem Solving through Programming In C 2022”
Program : 1 Write a C program which accept date, month and year as Input from the user, and using switch case, display the date in worded format.
Input: day=16, month=7, y=1992
Output: 16th July, 1992
#include
int main()
{
int month = 0;
int day = 0;
int year = 0;
scanf("%d", &day);
scanf("%d", &month);
scanf("%d", &year);
if(month>=1 && month <=12){
switch(month){
case 1:printf("%dth January %d",day,year);
break;
case 2:printf("%dth February %d",day,year);
break;
case 3:printf("%dth March %d",day,year);
break;
case 4:printf("%dth April %d",day,year);
break;
case 5:printf("%dth May %d",day,year);
break;
case 6:printf("%dth June %d",day,year);
break;
case 7:printf("%dth July %d",day,year);
break;
case 8:printf("%dth August %d",day,year);
break;
case 9:printf("%dth September %d",day,year);
break;
case 10:printf("%dth October %d",day,year);
break;
case 11:printf("%dth November %d",day,year);
break;
case 12:printf("%dth December %d",day,year);
break;
default: printf("Please type a valid number");
}
return 0;
}
}
Course Name : “Problem Solving through Programming In C 2022”
Program : 2 Write a C program to print a hollow rectangle pattern using “*” symbol. The number of rows and columns is accepted from the test case data.
For row = 5 and columns = 6 The output will be
#include
void hollow_rectangle(int n, int m);
int main()
{
int rows, columns;
scanf("%d", &rows);
scanf("%d", &columns);
hollow_rectangle(rows, columns);
return 0;
}
//Write the above function to print the hollow rectangle pattern
void hollow_rectangle(int n, int m)
{
int i, j;
for (i = 1; i <= n; i++)
{
for (j = 1; j <= m; j++)
{
if (i==1 || i==n || j==1 || j==m)
printf("*");
else
printf(" ");
}
printf("\n");
}
}
Programming Exam Oct 2022 (Second Session): (8PM - 9PM)
Course Name : “Problem Solving through Programming In C 2022”
Program : 1 Write a C program to replace a word in a given sentence with a given string and print the new sentence.
For example:
If the given sentence is : I like to play Tabla
Word to be replaced is : Tabla
Word to be replaced with: Guitar
The new sentence will be printed as: I like to play Guitar
#include
#include
void replaceSubstring(char [],char[],char[]);
int main()
{
char string[100],sub[100],new_str[100];
scanf("%[^\n]%*c", string); //Accept a sentence from test data
scanf("%[^\n]%*c", sub); //Accepts the word to be replaced
scanf("%[^\n]%*c", new_str ); //Accepts the word to be replaced with
replaceSubstring(string,sub,new_str);
printf("%s", string);
return 0;
}
void replaceSubstring(char string[],char sub[],char new_str[])
{
int stringLen,subLen,newLen;
int i=0,j,k;
int flag=0,start,end;
stringLen=strlen(string);
subLen=strlen(sub);
newLen=strlen(new_str);
for(i=0;i=j;k--)
string[k+1]=string[k];
string[j]=new_str[j-start];
stringLen++;
i++;
}
}
}
}
Course Name : “Problem Solving through Programming In C 2022”
Program : 2 Write a C program to print a triangle of prime numbers upto given number of lines of the triangle.
Example:
If number of lines is 3
the triangle will be
2
3 5
7 11 13
int prime(int num); //Function to find whether the number is prime or not.
int main() {
int lines;
scanf("%d", &lines);
int i,j,counter=2;
for(i = 1; i <= lines; i++)
{
for(j = 1; j <= i; j++)
{
while(!prime(counter))
{
counter++;
}
printf("%d\t", counter);
counter++;
}
printf("\n");
}
return(0);
}
int prime(int num)
{
int i, isPrime = 1;
for(i = 2; i <= (num/2); i++)
{
if(num % i == 0)
{
isPrime = 0;
break;
}
}
if(isPrime==1 || num==2)
return 1;
else
return 0;
}