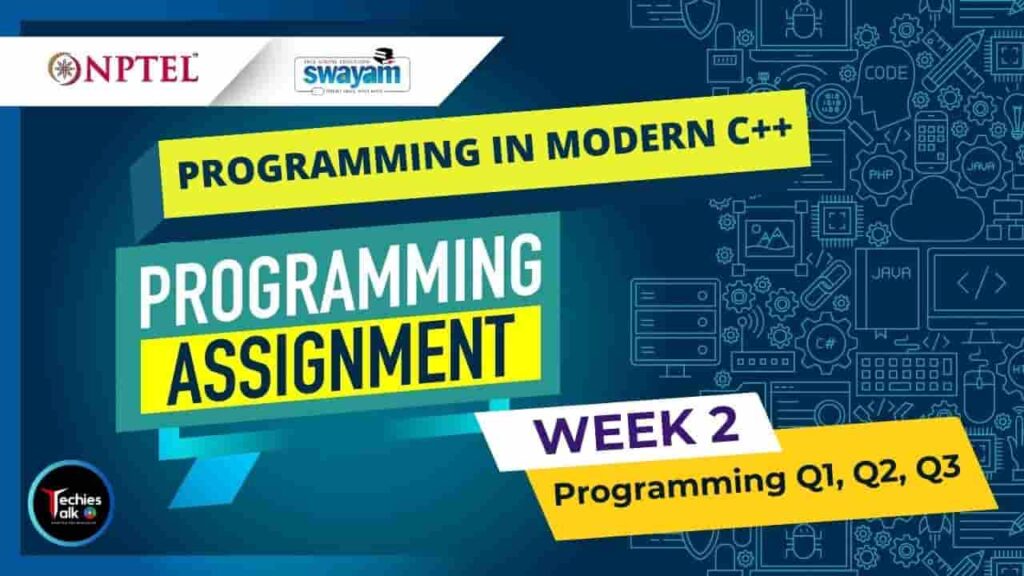
There has been a continual debate on which programming language/s to learn, to use. As the latest TIOBE Programming Community Index for August 2021 indicates – C (13%), Python (12%), C++ (7%), Java (10%), and C#(5%) together control nearly half the programming activities worldwide. Further, C Programming Language Family (C, C++, C#, Objective C etc.) dominate more than 25% of activities. Hence, learning C++ is important as one learns about the entire family, about Object-Oriented Programming and gets a solid foundation to also migrate to Java and Python as needed. C++ is the mother of most general purpose of languages. It is multi-paradigm encompassing procedural, object-oriented, generic, and even functional programming. C++ has primarily been the systems language till C++03 which punches efficiency of the code with the efficacy of OOP. Then, why should I learn it if my primary focus is on applications? This is where the recent updates of C++, namely, C++11 and several later offer excellent depths and flexibility for C++ that no language can match. These extensions attempt to alleviate some of the long-standing shortcomings for C++ including porous resource management, error-prone pointer handling, expression semantics, and better readability. The present course builds up on the knowledge of C programming and basic data structure (array, list, stack, queue etc.) to create a strong familiarity with C++98 / C++03. Besides the constructs, syntax and semantics of C++ (over C), we also focus on various idioms of C++ and attempt to go to depth with every C++ feature justifying and illustrating them with several examples and assignment problems. On the way, we illustrate various OOP concepts. The course also covers important advances in C++11 and later released features..
Programming In Modern C++ Week2 Programming Assignment 2023.
INTENDED AUDIENCE : Any interested audience
PREREQUISITES : 10th standard/high school
INDUSTRY SUPPORT : Programming in C++ is so fundamental that all companies dealing with systems as well as application development (including web, IoT, embedded systems) have a need for the same. These include – Microsoft, Samsung, Xerox, Yahoo, Oracle, Google, IBM, TCS, Infosys, Amazon, Flipkart, etc. This course would help industry developers to be up-to-date with the advances in C++ so that they can remain at the state-of-the-art.
Course Layout
Programming In Modern C++ 2023
Programming Assignment Q1 :
#include
using namespace std;
void print(int a, int b=0){ // LINE-1
int r = b + a;
cout << r;
}
int main() {
int a, b;
cin >> a >> b;
if (b < 0)
print(a);
else
print(a,b);
return 0;
}
Programming In Modern C++ 2023
Programming Assignment Q2 :
#include
using namespace std;
int Fun(int& x) { // LINE-1
x = x * x; // LINE-2
return x;
}
int main() {
int x, y;
cin >> x;
y = Fun(x);
cout << x << " " << y;
return 0;
}
Programming In Modern C++ 2023
Programming Assignment Q3 :
#include
using namespace std;
struct point {
int x, y;
};
point operator+(point &pt1, point &pt2){ //LINE-1
pt1.x += pt2.x;
pt1.y += pt2.y;
return pt1; //LINE-2
}
int main() {
int a, b, c, d;
cin >> a >> b >> c >> d;
point p1 = {a, b};
point p2 = {c, d};
point np = p1 + p2;
cout << "(" << p1.x << ", " << p1.y << ")" << "(" << np.x << ", " << np.y << ")";
return 0;
}