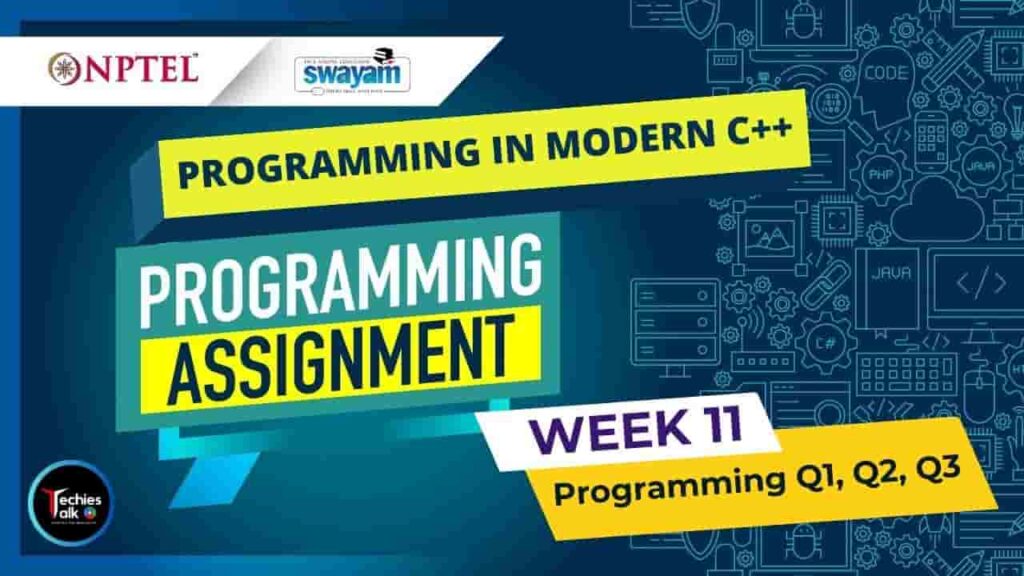
There has been a continual debate on which programming language/s to learn, to use. As the latest TIOBE Programming Community Index for August 2021 indicates – C (13%), Python (12%), C++ (7%), Java (10%), and C#(5%) together control nearly half the programming activities worldwide. Further, C Programming Language Family (C, C++, C#, Objective C etc.) dominate more than 25% of activities. Hence, learning C++ is important as one learns about the entire family, about Object-Oriented Programming and gets a solid foundation to also migrate to Java and Python as needed. C++ is the mother of most general purpose of languages. It is multi-paradigm encompassing procedural, object-oriented, generic, and even functional programming. C++ has primarily been the systems language till C++03 which punches efficiency of the code with the efficacy of OOP. Then, why should I learn it if my primary focus is on applications? This is where the recent updates of C++, namely, C++11 and several later offer excellent depths and flexibility for C++ that no language can match. These extensions attempt to alleviate some of the long-standing shortcomings for C++ including porous resource management, error-prone pointer handling, expression semantics, and better readability. The present course builds up on the knowledge of C programming and basic data structure (array, list, stack, queue etc.) to create a strong familiarity with C++98 / C++03. Besides the constructs, syntax and semantics of C++ (over C), we also focus on various idioms of C++ and attempt to go to depth with every C++ feature justifying and illustrating them with several examples and assignment problems. On the way, we illustrate various OOP concepts. The course also covers important advances in C++ 11 and later released features..
Programming In Modern C++ Week11 Programming Assignment July 2023
INTENDED AUDIENCE : Any interested audience
PREREQUISITES : 10th standard/high school
INDUSTRY SUPPORT : Programming in C++ is so fundamental that all companies dealing with systems as well as application development (including web, IoT, embedded systems) have a need for the same. These include – Microsoft, Samsung, Xerox, Yahoo, Oracle, Google, IBM, TCS, Infosys, Amazon, Flipkart, etc. This course would help industry developers to be up-to-date with the advances in C++ so that they can remain at the state-of-the-art.
Course Layout
Course Name : Programming In Modern C++ July 2023
Programming Assignment : Q1
#include
template //LINE-1
double product(T num){ return num; } //LINE-2
template //LINE-3
double product(T num, Tail... nums){
return num * product(nums...); //LINE-4
}
int main(){
int a, b, c;
double d, e, f;
std::cin >> a >> b >> c;
std::cin >> d >> e >> f;
std::cout << product(a, b, c) << " ";
std::cout << product(d, e, f) << " ";
std::cout << product(a, b, c, d, e, f);
return 0;
}
Course Name : Programming In Modern C++ July 2023
Programming Assignment : Q2
#include
class base {
public:
base(const int& n) : n_(n * 10){ std::cout << "lvalue : " << n << ", "; }
base(int&& n) : n_(n * 20) { std::cout << "rvalue : " << n << ", "; }
protected:
int n_;
};
class derived : public base {
public:
template //LINE-1
derived(T&& n) : base(std::forward(n)) { } //LINE-2
void show(){ std::cout << n_ << " "; }
};
int main(){
int i;
std::cin >> i;
derived obj1(i);
derived obj2(std::move(i));
obj1.show();
obj2.show();
return 0;
}
Course Name : Programming In Modern C++ July 2023
Programming Assignment : Q3
#include
#include
int main() {
std::function revPrint; //LINE-1
revPrint = [&revPrint](int n) -> int { //LINE-2
if (n == 0)
return 0;
return n % 10 + revPrint(n /= 10);
};
int a;
std::cin >> a;
std::cout << revPrint(a);
}