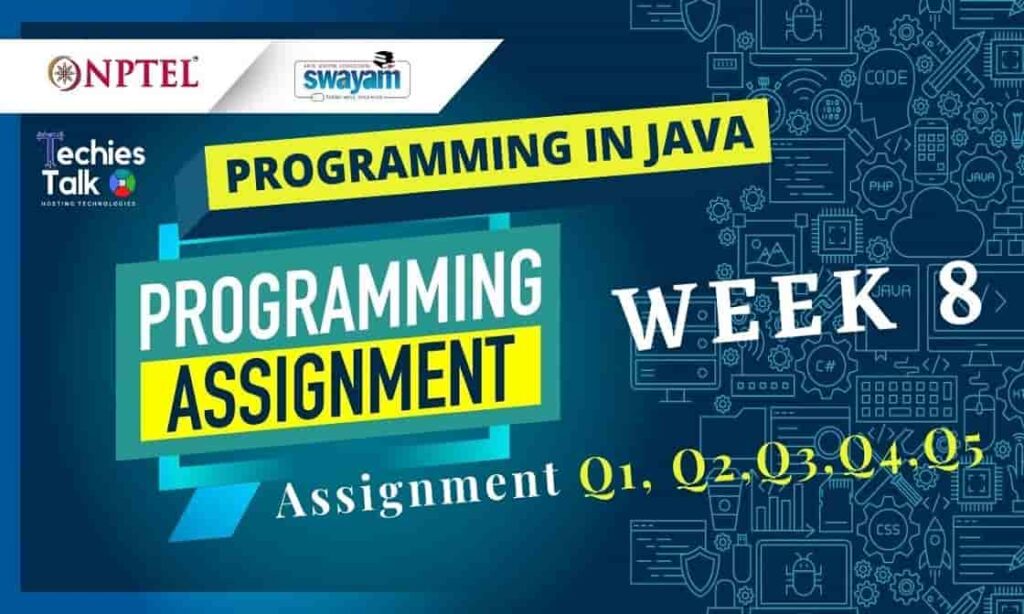
NPTEL Programming in Java Week 8 All Programming Assignment Solutions | Swayam. With the growth of Information and Communication Technology, there is a need to develop large and complex software.
Further, those software should be platform independent, Internet enabled, easy to modify, secure, and robust. To meet this requirement object-oriented paradigm has been developed and based on this paradigm the Java programming language emerges as the best programming environment.
Now, Java programming language is being used for mobile programming, Internet programming, and many other applications compatible to distributed systems.
This course aims to cover the essential topics of Java programming so that the participants can improve their skills to cope with the current demand of IT industries and solve many problems in their own filed of studies.
COURSE LAYOUT
- Week 1 : Overview of Object-Oriented Programming and Java
- Week 2 : Java Programming Elements
- Week 3 : Input-Output Handling in Java
- Week 4 : Encapsulation
- Week 5 : Inheritance
- Week 6 : Exception Handling
- Week 7 : Multithreaded Programming
- Week 8 : Java Applets and Servlets
- Week 9 : Java Swing and Abstract Windowing Toolkit (AWT)
- Week 10 : Networking with Java
- Week 11: Java Object Database Connectivity (ODBC)
- Week 12: Interface and Packages for Software Development
Course Name : “Programming in Java”
Question : 1 Write a program which will print a pyramid of “*” ‘s of height “n” and print the number of “*” ‘s in the pyramid.
import java.util.*;
public class Pattern1 {
public static void main(String[] args) {
Scanner inr = new Scanner(System.in);
int n = inr.nextInt();
int k = 0,sum=0;
for(int i = 1; i <= n; ++i, k = 0) {
for(int space = 1; space <= n - i; ++space) {
System.out.print(" ");
}
while(k != 2 * i - 1) {
System.out.print("* ");
sum=sum+1;
++k;
}
System.out.println();
}
System.out.println(sum);
}
}
Question : 2 Write a program which will print a pascal pyramid of “*” ‘s of height “l”.
import java.util.*;
public class Pattern2 {
public static void main(String[] args) {
Scanner inr = new Scanner(System.in);
int l = inr.nextInt();
int i,j;
int space=l-1;
/*run loop (parent loop) till number of rows*/
for(i=0;i< l;i++)
{
/*loop for initially space, before star printing*/
for(j=0;j< space;j++)
{
System.out.print(" ");
}
for(j=0;j<=i;j++)
{
System.out.print("* ");
}
System.out.print("\n");
space--; /* decrement one space after one row*/
}
}
}
Question : 3 Write a program which will print a pyramid of “numbers” ‘s of height “n” and print the sum of all number’s in the pyramid.
import java.util.*;
public class Pattern3 {
public static void main(String[] args) {
Scanner inr = new Scanner(System.in);
int n = inr.nextInt();
int k = 1,sum=0;
for(int i = 1; i <= n; ++i, k = 1) {
for(int space = 1; space <= n-i; ++space) {
System.out.print(" ");
}
while(k <= 2 * i - 1) {
System.out.print(k+" ");
sum=sum+k;
++k;
}
System.out.println();
}
System.out.println(sum);
}
}
Question : 4 Write a program to print symmetric Pascal’s triangle of “*” ‘s of height “l” of odd length . If input “l” is even then your program will print “Invalid line number”.
import java.util.*;
public class Pattern4 {
public static void main(String[] args) {
Scanner inr = new Scanner(System.in);
int l = inr.nextInt();
int ul=0; // Upper Line
int ll=0; // Lower Line
// Check whether line number is odd
if (l%2!=0){
ul=(l/2)+1;
ll=l-ul;
//Code for upper half
for(int i=1;i<=ul; i++){
//Space management
for(int s=1;s<=(ul-i); s++){
System.out.print(" ");
}
// Star management
for(int j=1;j<=i; j++){
System.out.print("* ");
}
System.out.println();
}
//Code for lower half
int llc=ll;
for(int i=1;i<=ll; i++){
//Space management
for(int s=llc;s
Question : 5 Write a program to display any digit(n) from 0-9 using “7 segment display“.
import java.util.*;
public class Pattern5 {
private static final Map encodings =
new HashMap();
static {
encodings.put(0, 0x7E);
encodings.put(1, 0x30);
encodings.put(2, 0x6D);
encodings.put(3, 0x79);
encodings.put(4, 0x33);
encodings.put(5, 0x5B);
encodings.put(6, 0x5F);
encodings.put(7, 0x70);
encodings.put(8, 0x7F);
encodings.put(9, 0x7B);
}
public static void printDigit(int digit) {
int code = encode(digit);
char[] bits =
String.format("%7s", Integer.toBinaryString(code))
.replace(' ', '0').toCharArray();
lightSegment(bits[0] == '1', " _ \n", " \n");
lightSegment(bits[5] == '1', "|", " ");
lightSegment(bits[6] == '1', "_", " ");
lightSegment(bits[1] == '1', "|\n", " \n");
lightSegment(bits[4] == '1', "|", " ");
lightSegment(bits[3] == '1', "_", " ");
lightSegment(bits[2] == '1', "|\n", " \n");
}
private static void lightSegment(boolean on, String onValue, String offValue) {
System.out.print(on ? onValue : offValue);
try {
Thread.sleep(0);
}
catch (InterruptedException e) {
e.printStackTrace();
}
}
private static int encode(int digit) {
return encodings.containsKey(digit) ? encodings.get(digit) : 0x00;
}
public static void main(String[] args) throws Exception {
Scanner inr = new Scanner(System.in);
int n = inr.nextInt();
printDigit(n);
}
}