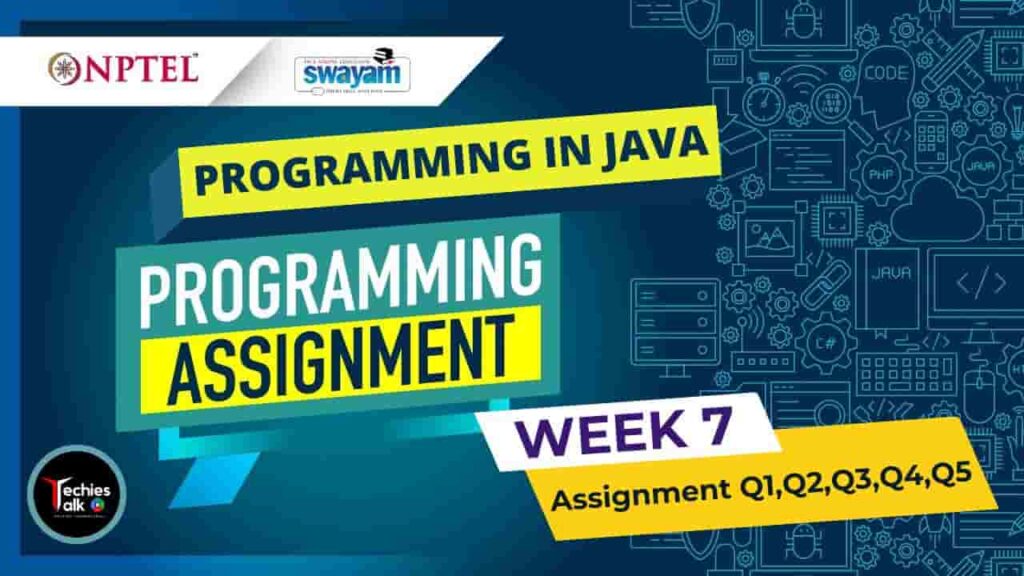
NPTEL Programming in Java Week 7 All Programming Assignment Solutions – Jan 2023 | Swayam. With the growth of Information and Communication Technology, there is a need to develop large and complex software.
Further, those software should be platform independent, Internet enabled, easy to modify, secure, and robust. To meet this requirement object-oriented paradigm has been developed and based on this paradigm the Java programming language emerges as the best programming environment.
Now, Java programming language is being used for mobile programming, Internet programming, and many other applications compatible to distributed systems.
This course aims to cover the essential topics of Java programming so that the participants can improve their skills to cope with the current demand of IT industries and solve many problems in their own filed of studies.
COURSE LAYOUT
- Week 1 : Overview of Object-Oriented Programming and Java
- Week 2 : Java Programming Elements
- Week 3 : Input-Output Handling in Java
- Week 4 : Encapsulation
- Week 5 : Inheritance
- Week 6 : Exception Handling
- Week 7 : Multithreaded Programming
- Week 8 : Java Applets and Servlets
- Week 9 : Java Swing and Abstract Windowing Toolkit (AWT)
- Week 10 : Networking with Java
- Week 11: Java Object Database Connectivity (ODBC)
- Week 12: Interface and Packages for Software Development
Course Name : “Programming in Java 2023”
Question : 1 A byte char array is initialized. You have to enter an index value”n“. According to index your program will print the byte and its corresponding char value.
Complete the code segment to catch the exception in the following, if any. On the occurrence of such an exception, your program should print “exception occur” .If there is no such exception, it will print the required output.
import java.util.*;
public class Question3 {
public static void main(String[] args) {
try{
byte barr[]={'N','P','T','E','L','-','J','A','V','A','J','A','N','-','N','O','C','C','S','E'};
Scanner inr = new Scanner(System.in);
int n = inr.nextInt();
String s2 = new String(barr,n,1);
System.out.println(barr[n]);
System.out.print(s2);
}
catch (Exception e){
System.out.println("exception occur");
}
}
}
Question : 2 The following program reads a string from the keyboard and is stored in the String variable “s1“. You have to complete the program so that it should should print the number of vowels in s1 . If your input data doesn’t have any vowel it will print “0“.
import java.io.*;
import java.util.*;
public class Question4{
public static void main(String[] args) {
int c=0;
try{
InputStreamReader r=new InputStreamReader(System.in);
BufferedReader br=new BufferedReader(r);
String s1 = br.readLine();
//Write your code here to count the number of vowels in the string "s1"
for(int i=0;i
Question : 3 A string “s1” is already initialized. You have to read the index “n” from the keyboard. Complete the code segment to catch the exception in the following, if any. On the occurrence of such an exception, your program should print “exception occur” .If there is no such exception, your program should replace the char “a” at the index value “n” of the “s1” ,then it will print the modified string.
import java.util.*;
public class Question5 {
public static void main(String[] args) {
try{
String s1="NPTELJAVA";
Scanner inr = new Scanner(System.in);
int n = inr.nextInt();
char c='a';
//Replace the char in string "s1" with the char 'a' at index "n" and print the modified string
byte[] barr=s1.getBytes();
byte b1 = (byte) c;
barr[n]=b1;
System.out.print(new String(barr));
}
catch (Exception e){
System.out.println("exception occur");
}
}
}
Question : 4 Complete the following code fragment to read three integer values from the keyboard and find the sum of the values. Declare a variable “sum” of type int and store the result in it.
// Write the appropriate statement(s) to import the package(s) you need in your program
import java.util.*;
public class Question1{
public static void main (String[] args){
//Write the appropriate code to read the 3 integer values and find their sum.
int i,n=0,sum=0;
Scanner in = new Scanner(System.in);
for(i=0;i<3;i++)
{
n = in.nextInt();
sum =sum+n;
}
System.out.println(sum);
}
}
Question : 5 Complete the code segment to catch the exception in the following, if any. On the occurrence of such an exception, your program should print “Please enter valid data” .If there is no such exception, it will print the “square of the number“.
import java.io.*;
public class Question2{
public static void main(String args[]){
//Use appropriate Try..catch block to complete the code
try{
InputStreamReader r=new InputStreamReader(System.in);
BufferedReader br=new BufferedReader(r);
String number=br.readLine();
int x = Integer.parseInt(number);
System.out.print(x*x);
}
catch(Exception e){
System.out.print("Please enter valid data");
}
}
}