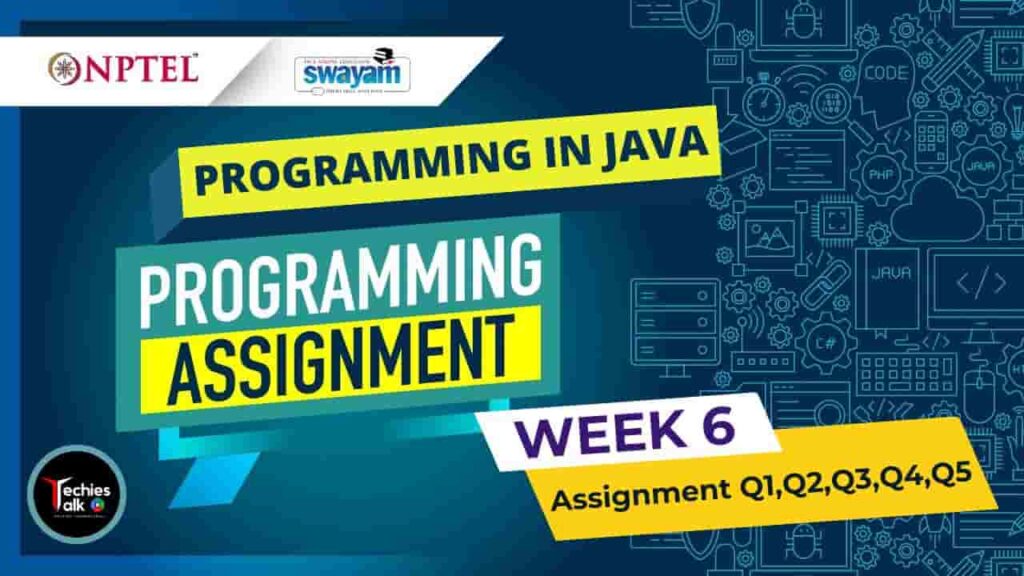
NPTEL Programming in Java Week 6 All Programming Assignment Solutions – Jan 2023 | Swayam. With the growth of Information and Communication Technology, there is a need to develop large and complex software.
Further, those software should be platform independent, Internet enabled, easy to modify, secure, and robust. To meet this requirement object-oriented paradigm has been developed and based on this paradigm the Java programming language emerges as the best programming environment.
Now, Java programming language is being used for mobile programming, Internet programming, and many other applications compatible to distributed systems.
This course aims to cover the essential topics of Java programming so that the participants can improve their skills to cope with the current demand of IT industries and solve many problems in their own filed of studies.
COURSE LAYOUT
- Week 1 : Overview of Object-Oriented Programming and Java
- Week 2 : Java Programming Elements
- Week 3 : Input-Output Handling in Java
- Week 4 : Encapsulation
- Week 5 : Inheritance
- Week 6 : Exception Handling
- Week 7 : Multithreaded Programming
- Week 8 : Java Applets and Servlets
- Week 9 : Java Swing and Abstract Windowing Toolkit (AWT)
- Week 10 : Networking with Java
- Week 11: Java Object Database Connectivity (ODBC)
- Week 12: Interface and Packages for Software Development
Course Name : “Programming in Java 2023”
Question : 1 Complete the code segment to print the following using the concept of extending the Thread class in Java:
—————–OUTPUT——————-
Thread is Running.
————————————————-
// Write the appropriate code to extend the Thread class to complete the class Question61.
public class Question61 extends Thread
{
public void run()
{
System.out.print("Thread is Running.");
}
public static void main(String args[]){
// Creating object of thread class
Question61 thread=new Question61();
// Start the thread
thread.start();
}
}
Question : 2 In the following program, a thread class Question62 is created using the Runnable interface Complete the main() to create a thread object of the class Question62 and run the thread. It should print the output as given below.
—————–OUTPUT——————-
Welcome to Java Week 6 New Question.
Main Thread has ended.
————————————————-
public class Question62 implements Runnable {
@Override
public void run() {
System.out.print(Thread.currentThread().getName()+" has ended.");
}
// Create main() method and appropriate statements in it
public static void main(String[] args)
{
Question62 ex = new Question62();
Thread t1= new Thread(ex);
t1.setName("Main Thread");
t1.start();
System.out.println("Welcome to Java Week 6 New Question.");
t1.setName("Main Thread");
}
}
Question : 3 A part of the Java program is given, which can be completed in many ways, for example using the concept of thread, etc. Follow the given code and complete the program so that your program prints the message “NPTEL Java week-6 new Assignment Q3“. Your program should utilize the given interface/ class.Invalid HTML tag: tag name o:p is not allowed
// Interface A is defined with an abstract method run()
interface A {
public abstract void run();
}
// Class B is defined which implements A and an empty implementation of run()
class B implements A {
public void run() {}
}
// Create a class named MyThread and extend/implement the required class/interface
class MyThread extends B
{
// Define a method in MyThread class to print the output
public void run()
{
System.out.print("NPTEL Java week-6 new Assignment Q3");
}
}
// Main class Question is defined
public class Question63 {
public static void main(String[] args) {
// An object of MyThread class is created
MyThread t = new MyThread();
// run() of class MyThread is called
t.run();
}
}
Question : 4 Execution of two or more threads occurs in a random order. The keyword ‘synchronized’ in Java is used to control the execution of thread in a strict sequence. In the following, the program is expected to print the output as given below. Do the necessary use of ‘synchronized’ keyword, so that, the program prints the Final sum as given below:
—————–OUTPUT——————-
Final sum:6000
————————————————-
class Pair {
private int a, b;
public Pair() {
a = 0;
b = 0;
}
// Returns the sum of a and b. (reader)
// Should always return an even number.
public synchronized int sum()
{
return(a+b);
}
// Increments both a and b. (writer)
public synchronized void inc()
{
a++;
b++;
}
}
public class PairWorker extends Thread {
public final int COUNT = 1000;
private Pair pair;
// Ctor takes a pointer to the pair we use
public PairWorker(Pair pair) {
this.pair = pair;
}
// Send many inc() messages to our pair
public void run() {
for(int i=0; i
Question : 5 Given a snippet of code, add necessary codes to print the following:
—————–OUTPUT——————-
Name of thread ‘t1’:Thread-0
Name of thread ‘t2’:Thread-1
New name of thread ‘t1’:Week 6 Assignment Q5
New name of thread ‘t2’:Week 6 Assignment Q5 New
————————————————-
public class Question65 extends Thread{
public void run(){
}
public static void main(String args[]){
Question65 t1=new Question65();
System.out.println("Name of thread 't1':"+ t1.getName());
Question65 t2=new Question65();
System.out.println("Name of thread 't2':"+ t2.getName());
// start the thread-1
t1.start();
// set the name of thread-1
t1.setName("Week 6 Assignment Q5");
// start the thread-2
t2.start();
// set the name of thread-2
t2.setName("Week 6 Assignment Q5 New");
System.out.println("New name of thread 't1':"+ t1.getName());
System.out.println("New name of thread 't2':"+ t2.getName());
}
}