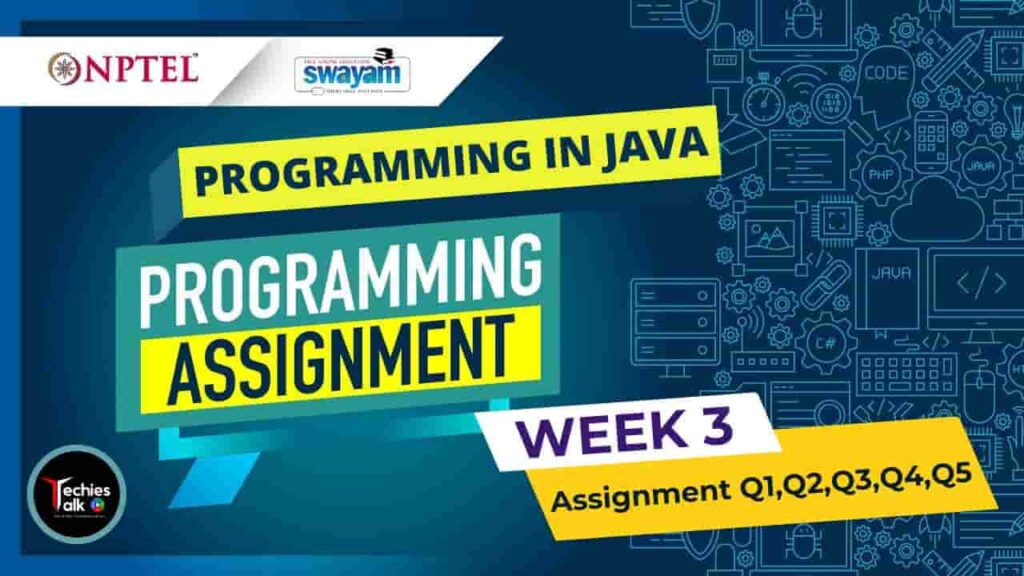
NPTEL Programming in Java Week3 All Programming Assignment Solutions – Jan 2023 | Swayam. With the growth of Information and Communication Technology, there is a need to develop large and complex software.
Further, those software should be platform independent, Internet enabled, easy to modify, secure, and robust. To meet this requirement object-oriented paradigm has been developed and based on this paradigm the Java programming language emerges as the best programming environment.
Now, Java programming language is being used for mobile programming, Internet programming, and many other applications compatible to distributed systems.
This course aims to cover the essential topics of Java programming so that the participants can improve their skills to cope with the current demand of IT industries and solve many problems in their own filed of studies.
COURSE LAYOUT
-
- Week 1 : Overview of Object-Oriented Programming and Java
- Week 2 : Java Programming Elements
- Week 3 : Input-Output Handling in Java
- Week 4 : Encapsulation
- Week 5 : Inheritance
- Week 6 : Exception Handling
- Week 7 : Multithreaded Programming
- Week 8 : Java Applets and Servlets
- Week 9 : Java Swing and Abstract Windowing Toolkit (AWT)
- Week 10 : Networking with Java
- Week 11: Java Object Database Connectivity (ODBC)
- Week 12: Interface and Packages for Software Development
Course Name : “Programming in Java 2023”
Question : 1 This program is related to the generation of Fibonacci numbers.
For example: 0,1, 1,2, 3,5, 8, 13,… is a Fibonacci sequence where 13 is the 8th Fibonacci number.
import java.util.Scanner;
public class Fibonacci {
public static void main(String args[]){
Scanner sc = new Scanner(System.in);
int n=sc.nextInt();
System.out.println(fib(n));
}
//Template code:
static int fib(int n) {
if (n==1) //Terminal condition
return 0;
else if(n==2)
return 1;
return fib(n - 1) + fib(n - 2); //Recursive call of function
}
}
Question : 2 Define a class Point with two fields x and y each of type double. Also, define a method distance(Point p1, Point p2) to calculate the distance between points p1 and p2 and return the value in double.
import java.util.Scanner;
public class Circle extends Point{
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
Point p1=new Point();
p1.x=sc.nextDouble();
p1.y=sc.nextDouble();
Point p2=new Point();
p2.x=sc.nextDouble();
p2.y=sc.nextDouble();
Circle c1=new Circle();
c1.distance(p1,p2);
}
}
class Point{
double x;
double y;
public static void distance(Point p1,Point p2)
{
double d;
d=Math.sqrt((p2.x-p1.x)*(p2.x-p1.x) + (p2.y-p1.y)*(p2.y-p1.y));
System.out.print(d);
}
}
Question : 3 A class Shape is defined with two overloading constructors in it. Another class Test1 is partially defined which inherits the class Shape. The class Test1 should include two overloading constructors as appropriate for some object instantiation shown in main() method. You should define the constructors using the super class constructors. Also, override the method calculate( ) in Test1 to calculate the volume of a Shape.
import java.util.Scanner;
class Shape{
double length, breadth;
Shape(double l, double b){ //Constructor to initialize a Shape object
length = l;
breadth= b;
}
Shape(double len){ //Constructor to initialize another Shape object
length = breadth = len;
}
double calculate(){// To calculate the area of a shape object
return length * breadth ;
}
}
public class Test1 extends Shape{
//Template code:
double height;
Test1(double length,double h) {
//base class constructor with one parameter is called
super(length);
height=h;
}
Test1(double length,double breadth,double h) {
//base class constructor having two argument is called
super(length,breadth);
height=h;
}
double calculate() {
return length*breadth*height;
}
public static void main(String args[]){
Scanner sc = new Scanner(System.in);//Create an object to read
//input
double l=sc.nextDouble(); //Read length
double b=sc.nextDouble(); //Read breadth
double h=sc.nextDouble(); //Read height
Test1 myshape1 = new Test1(l,h);
Test1 myshape2 = new Test1(l,b,h);
double volume1;
double volume2;
volume1 = myshape1.calculate();
volume2=myshape2.calculate();
System.out.println(volume1);
System.out.println(volume2);
}
}
Question : 4 This program to exercise the call of static and non-static methods. A partial code is given defining two methods, namely sum( ) and multiply ( ). You have to call these methods to find the sum and product of two numbers. Complete the code segment as instructed.
import java.util.Scanner;
class QuestionScope {
int sum(int a, int b){ //non-static method
return a + b;
}
static int multiply(int a, int b){ //static method
return a * b;
}
}
public class Test3{
public static void main( String[] args ) {
Scanner sc = new Scanner(System.in);
int n1=sc.nextInt();
int n2=sc.nextInt();
//Called the method sum() to find the sum of two numbers.
//Called the method multiply() to find the product of two numbers.
QuestionScope st = new QuestionScope(); // Create an object to call non-static method
int result1=st.sum(n1,n2); // Call the method
int result2=QuestionScope.multiply(n1,n2); // Create an object to call static method
System.out.println(result1);
System.out.print(result2);
}
}
Question : 5 Complete the code segment to swap two numbers using call by object reference.
import java.util.Scanner;
class Question { //Define a class Question with two elements e1 and e2.
Scanner sc = new Scanner(System.in);
int e1 = sc.nextInt(); //Read e1
int e2 = sc.nextInt(); //Read e2
}
public class Question3 {
// Define static method swap()to swap the values of e1 and e2 of class Question.
public static void swap(Question t)
{
int temp = t.e1;
t.e1 = t.e2;
t.e2 = temp;
}
public static void main(String[] args) {
//Create an object of class Question
Question t = new Question ();
//Call the method swap()
swap(t);
System.out.println(t.e1+" "+t.e2);
}
}