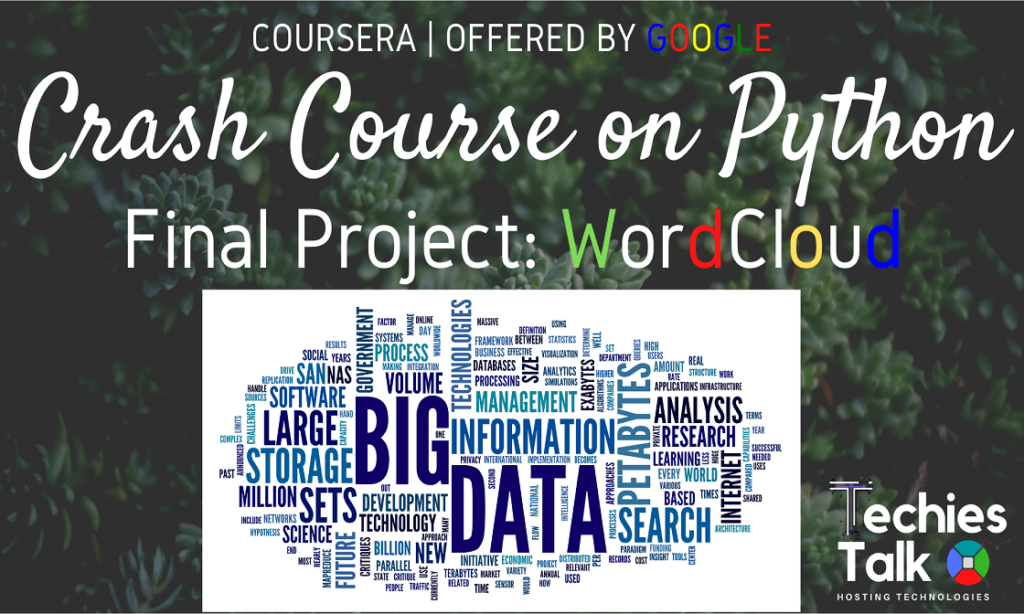
This course is designed to teach you the foundations in order to write simple programs in Python using the most common structures. This is crash course on python coursera final project – wordcloud.
No previous exposure to programming is needed. By the end of this course, you’ll understand the benefits of programming in IT roles; be able to write simple programs using Python; figure out how the building blocks of programming fit together; and combine all of this knowledge to solve a complex programming problem.
We’ll start off by diving into the basics of writing a computer program. Along the way, you’ll get hands-on experience with programming concepts through interactive exercises and real-world examples. You’ll quickly start to see how computers can perform a multitude of tasks — you just have to write code that tells them what to do.
~~:WHAT YOU WILL LEARN:~~
- Understand what Python is and why Python is relevant to automation
- Write short Python scripts to perform automated actions
- Understand how to use the basic Python structures: strings, lists, and dictionaries
- Create your own Python objects
Final Project – Word Cloud
For this project, you’ll create a “word cloud” from a text by writing a script. This script needs to process the text, remove punctuation, ignore case and words that do not contain all alphabets, count the frequencies, and ignore uninteresting or irrelevant words. A dictionary is the output of the calculate_frequencies
function. The wordcloud
module will then generate the image from your dictionary.
For the input text of your script, you will need to provide a file that contains text only. For the text itself, you can copy and paste the contents of a website you like. Or you can use a site like Project Gutenberg to find books that are available online. You could see what word clouds you can get from famous books, like a Shakespeare play or a novel by Jane Austen. Save this as a .txt file somewhere on your computer.
Now you will need to upload your input file here so that your script will be able to process it. To do the upload, you will need an uploader widget. Run the following cell to perform all the installs and imports for your word cloud script and uploader widget. It may take a minute for all of this to run and there will be a lot of output messages. But, be patient. Once you get the following final line of output, the code is done executing. Then you can continue on with the rest of the instructions for this notebook.
Enabling notebook extension fileupload/extension…
– Validating: OK
# Here are all the installs and imports you will need for your word cloud script and uploader widget
!pip install wordcloud
!pip install fileupload
!pip install ipywidgets
!jupyter nbextension install --py --user fileupload
!jupyter nbextension enable --py fileupload
import wordcloud
import numpy as np
from matplotlib import pyplot as plt
from IPython.display import display
import fileupload
import io
import sys
Whew! That was a lot. All of the installs and imports for your word cloud script and uploader widget have been completed.
IMPORTANT! If this was your first time running the above cell containing the installs and imports, you will need save this notebook now. Then under the File menu above, select Close and Halt. When the notebook has completely shut down, reopen it. This is the only way the necessary changes will take affect.
To upload your text file, run the following cell that contains all the code for a custom uploader widget. Once you run this cell, a “Browse” button should appear below it. Click this button and navigate the window to locate your saved text file.
# This is the uploader widget
def _upload():
_upload_widget = fileupload.FileUploadWidget()
def _cb(change):
global file_contents
decoded = io.StringIO(change['owner'].data.decode('utf-8'))
filename = change['owner'].filename
print('Uploaded `{}` ({:.2f} kB)'.format(
filename, len(decoded.read()) / 2 **10))
file_contents = decoded.getvalue()
_upload_widget.observe(_cb, names='data')
display(_upload_widget)
_upload()
The uploader widget saved the contents of your uploaded file into a string object named file_contents that your word cloud script can process. This was a lot of preliminary work, but you are now ready to begin your script.
Write a function in the cell below that iterates through the words in file_contents, removes punctuation, and counts the frequency of each word. Oh, and be sure to make it ignore word case, words that do not contain all alphabets and boring words like “and” or “the”. Then use it in the generate_from_frequencies
function to generate your very own word cloud!
Hint: Try storing the results of your iteration in a dictionary before passing them into wordcloud via the generate_from_frequencies
function.
def calculate_frequencies(file_contents):
# Here is a list of punctuations and uninteresting words you can use to process your text
punctuations = '''!()-[]{};:'"\,<>./?@#$%^&*_~'''
uninteresting_words = ["the", "a", "to", "if", "is", "it", "of", "and", "or", "an", "as", "i", "me", "my", \
"we", "our", "ours", "you", "your", "yours", "he", "she", "him", "his", "her", "hers", "its", "they", "them", \
"their", "what", "which", "who", "whom", "this", "that", "am", "are", "was", "were", "be", "been", "being", \
"have", "has", "had", "do", "does", "did", "but", "at", "by", "with", "from", "here", "when", "where", "how", \
"all", "any", "both", "each", "few", "more", "some", "such", "no", "nor", "too", "very", "can", "will", "just"]
# LEARNER CODE START HERE
frequencies={}
file_contents=file_contents.split()
str1=""
for word in file_contents:
str1= ''.join(ch for ch in word if ch.isalnum())
if str1.lower() not in uninteresting_words:
if str1.lower() not in frequencies:
frequencies[str1.lower()]=1
else:
frequencies[str1.lower()]+=1
cloud = wordcloud.WordCloud()
cloud.generate_from_frequencies(frequencies)
return cloud.to_array()
If you have done everything correctly, your word cloud image should appear after running the cell below. Fingers crossed!
# Display your wordcloud image
myimage = calculate_frequencies(file_contents)
plt.imshow(myimage, interpolation = 'nearest')
plt.axis('off')
plt.show()
If your word cloud image did not appear, go back and rework your calculate_frequencies function until you get the desired output. Definitely check that you passed your frequecy count dictionary into the generate_from_frequencies function of wordcloud. Once you have correctly displayed your word cloud image, you are all done with this project. Nice work!
on the last bit, if everything is done correctly, I just have to run the cell. I run the cell and I get this error
—————————————————————————
NameError Traceback (most recent call last)
in
1 # Display your wordcloud image
2
—-> 3 myimage = calculate_frequencies(file_contents)
4 plt.imshow(myimage, interpolation = ‘nearest’)
5 plt.axis(‘off’)
NameError: name ‘file_contents’ is not defined