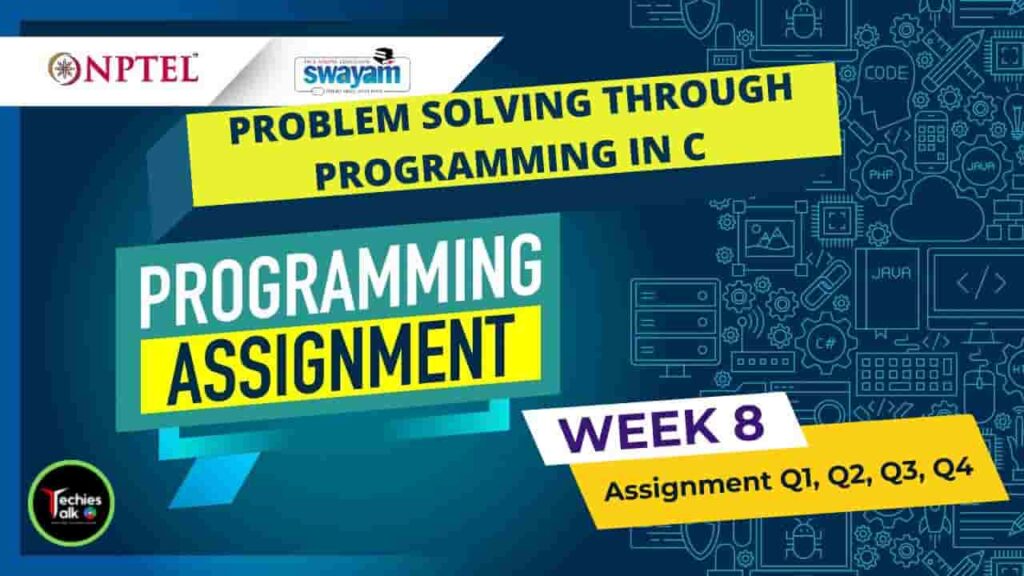
NPTEL Problem solving through Programming In C Week 8 All Programming Assignment Solutions | Swayam 2023. With the growth of Information and Communication Technology, there is a need to develop large and complex software.
ABOUT THE COURSE:
- Formulate simple algorithms for arithmetic and logical problems
- Translate the algorithms to programs (in C language)
- Test and execute the programs and correct syntax and logical errors
- Implement conditional branching, iteration and recursion
- Decompose a problem into functions and synthesize a complete program using divide and conquer approach
- Use arrays, pointers and structures to formulate algorithms and programs
- Apply programming to solve matrix addition and multiplication problems and searching and sorting problems
- Apply programming to solve simple numerical method problems, namely rot finding of function, differentiation of function and simple integration
COURSE LAYOUT
- Week 1 : Introduction to Problem Solving through programs, Flowcharts/Pseudo codes, the compilation process, Syntax and Semantic errors, Variables and Data Types
- Week 2 : Arithmetic expressions, Relational Operations, Logical expressions; Introduction to Conditional Branching
- Week 3 : Conditional Branching and Iterative Loops
- Week 4 : Arranging things : Arrays
- Week 5 : 2-D arrays, Character Arrays and Strings
- Week 6 : Basic Algorithms including Numerical Algorithms
- Week 7 : Functions and Parameter Passing by Value
- Week 8 : Passing Arrays to Functions, Call by Reference
- Week 9 : Recursion
- Week 10 : Structures and Pointers
- Week 11 : Self-Referential Structures and Introduction to Lists
- Week 12 : Advanced Topics
Once again, thanks for your interest in our online courses and certification. Happy learning!
Course Name : “Problem Solving through Programming In C 2023”
Question : 1 Write a C Program to find HCF of 4 given numbers using recursive function
#include
int HCF(int, int); //You have to write this function which calculates the HCF.
int main()
{
int a, b, c, d, result;
scanf("%d %d %d %d", &a, &b, &c, &d); /* Takes 4 number as input from the test data */
result = HCF(HCF(a, b), HCF(c,d));
printf("The HCF is %d", result);
}
int HCF(int x, int y)
{
while (x != y)
{
if (x > y)
return HCF(x - y, y);
else
return HCF(x, y - x);
}
return x;
}
Course Name : “Problem Solving through Programming In C 2023”
Question : 2 Write a C Program to find power of a given number using recursion. The number and the power to be calculated is taken from test case
#include
long power(int, int);
int main()
{
int pow, num;
long result;
scanf("%d", &num); //The number taken as input from test case data
scanf("%d", &pow); //The power is taken from the test case
result = power(num, pow);
printf("%d^%d is %ld", num, pow, result);
return 0;
}
long power(int n, int p)
{
if (p)
{
return (n * power(n, p - 1));
}
return(1);
}
Course Name : “Problem Solving through Programming In C 2023”
Question : 3 Write a C Program to print Binary Equivalent of an Integer using Recursion
#include
int binary_conversion(int);
int main()
{
int num, bin;
scanf("%d", &num);
bin = binary_conversion(num);
printf("The binary equivalent of %d is %d\n", num, bin);
return 0;
}
int binary_conversion(int num)
{
if(num == 0)
return 0;
else
return (num%2) + 10 * binary_conversion(num/2);
}
Course Name : “Problem Solving through Programming In C 2023”
Question : 4 Write a C program to print a triangle of prime numbers upto given number of lines of the triangle.
e.g If number of lines is 3 the triangle will be
2
3 5
7 11 13
#include
int prime(int num); //Function to find whether the number is prime or not.
int main() {
int lines;
scanf("%d", &lines);
int q, t;
int num = 2;
for (q = 0; q < lines; q++)
{
for (t = 0; t <= q; t++)
{
while (!prime(num))
{
num++;
}
printf("%d\t", num++);
}
printf("\n");
}
return (0);
}
int prime(int num)
{
int q, flag;
for (q = 2; q < num; q++)
{
if (num % q != 0)
flag = 1;
else {
flag = 0;
break;
}
}
if (flag == 1 || num == 2)
return (1);
else
return (0);
}