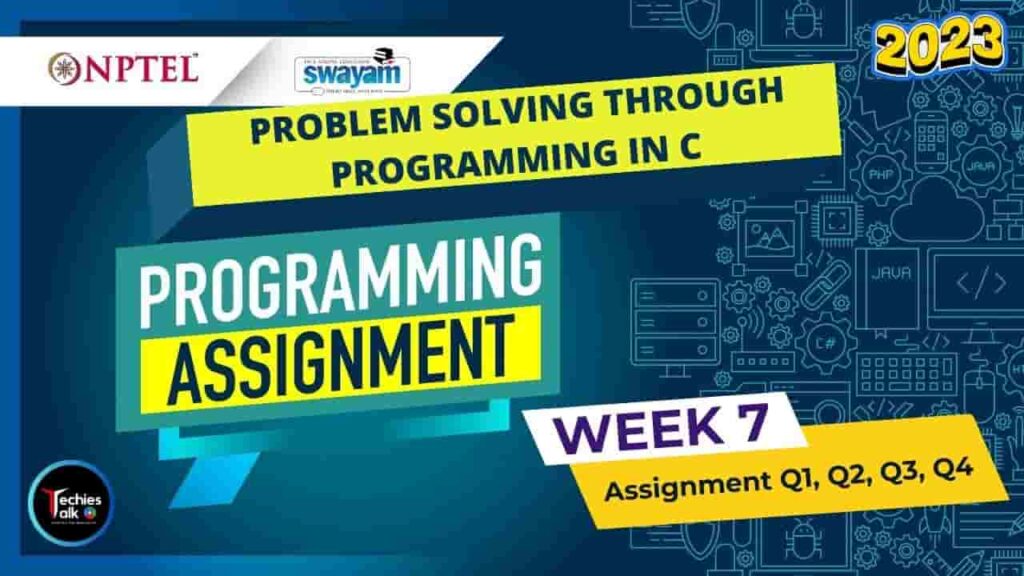
NPTEL Problem solving through Programming In C Week7 All Programming Assignment Solutions | Swayam July 2023. With the growth of Information and Communication Technology, there is a need to develop large and complex software.
ABOUT THE COURSE:
- Formulate simple algorithms for arithmetic and logical problems
- Translate the algorithms to programs (in C language)
- Test and execute the programs and correct syntax and logical errors
- Implement conditional branching, iteration and recursion
- Decompose a problem into functions and synthesize a complete program using divide and conquer approach
- Use arrays, pointers and structures to formulate algorithms and programs
- Apply programming to solve matrix addition and multiplication problems and searching and sorting problems
- Apply programming to solve simple numerical method problems, namely rot finding of function, differentiation of function and simple integration
COURSE LAYOUT
- Week 1 : Introduction to Problem Solving through programs, Flowcharts/Pseudo codes, the compilation process, Syntax and Semantic errors, Variables and Data Types
- Week 2 : Arithmetic expressions, Relational Operations, Logical expressions; Introduction to Conditional Branching
- Week 3 : Conditional Branching and Iterative Loops
- Week 4 : Arranging things : Arrays
- Week 5 : 2-D arrays, Character Arrays and Strings
- Week 6 : Basic Algorithms including Numerical Algorithms
- Week 7 : Functions and Parameter Passing by Value
- Week 8 : Passing Arrays to Functions, Call by Reference
- Week 9 : Recursion
- Week 10 : Structures and Pointers
- Week 11 : Self-Referential Structures and Introduction to Lists
- Week 12 : Advanced Topics
Once again, thanks for your interest in our online courses and certification. Happy learning!
Problem Solving through Programming In C July 2023
Question : 1 Write a C Program to Count Number of Uppercase and Lowercase Letters in a given string. The given string may be a word or a sentence.
#include
int main() {
int upper = 0, lower = 0;
char ch[100];
scanf(" %[^\n]s", ch);
int i = 0;
while (ch[i] != '\0')
{
if (ch[i] >= 'A' && ch[i] <= 'Z')
upper++;
if (ch[i] >= 'a' && ch[i] <= 'z')
lower++;
i++;
}
printf("Uppercase Letters : %d\n", upper); /*prints number of uppercase letters */
printf("Lowercase Letters : %d", lower); /*prints number of lowercase letters */
return (0);
}
Problem Solving through Programming In C July 2023
Question : 2 Write a C program to find the sum of all elements of each row of a matrix.
Example: For a matrix 4 5 6
6 7 3
1 2 3
The output will be : 15
16
6
#include
int main()
{
int matrix[20][20];
int i,j,r,c;
scanf("%d",&r); //Accepts number of rows
scanf("%d",&c); //Accepts number of columns
for(i=0;i< r;i++) //Accepts the matrix elements from the test case data
{
for(j=0;j< c;j++)
{
scanf("%d",&matrix[i][j]);
}
}
int sum;
for(i=0;i< r;i++)
{
sum=0;
for(j=0;j< c;j++)
{
// printf("%d\t",matrix[i][j]);
sum += matrix[i][j];
}
printf("%d\n",sum);
}
}
Problem Solving through Programming In C July 2023
Question : 3 Write a C program to find subtraction of two matrices i.e. matrix_A – matrix_B=matrix_C.
If the given martix are :
2 3 5 and 1 5 2 Then the output will be 1 -2 3
4 5 6 2 3 4 2 2 2
6 5 7 3 3 4 3 2 3
The elements of the output matrix are separated by one blank space.
#include
int main()
{
int matrix_A[20][20], matrix_B[20][20], matrix_C[20][20];
int i,j,row,col;
scanf("%d",&row); //Accepts number of rows
scanf("%d",&col); //Accepts number of columns
/* Elements of first matrix are accepted from test data */
for(i=0; i
Problem Solving through Programming In C July 2023
Question : 4 Write a C program to print Largest and Smallest Word from a given sentence. If there are two or more words of same length, then the first one is considered. A single letter in the sentence is also consider as a word.
#include
#include
int main()
{
char str[100]={0},substr[100][100]={0};
scanf("%[^\n]s", str);
int i=0,j=0,k=0,a,minIndex=0,maxIndex=0,max=0,min=0;
char c;
while(str[k]!='\0') //for splitting sentence into words
{
j=0;
while(str[k]!=' '&&str[k]!='\0' && str[k]!='.')
{
substr[i][j]=str[k];
k++;
j++;
}
substr[i][j]='\0';
i++;
if(str[k]!='\0')
{
k++;
}
}
int len=i;
max=strlen(substr[0]);
min=strlen(substr[0]);
//After splitting getting length of string and finding its index having max length and index having min length
for(i=0;imax)
{
max=a;
maxIndex=i;
}
if(a