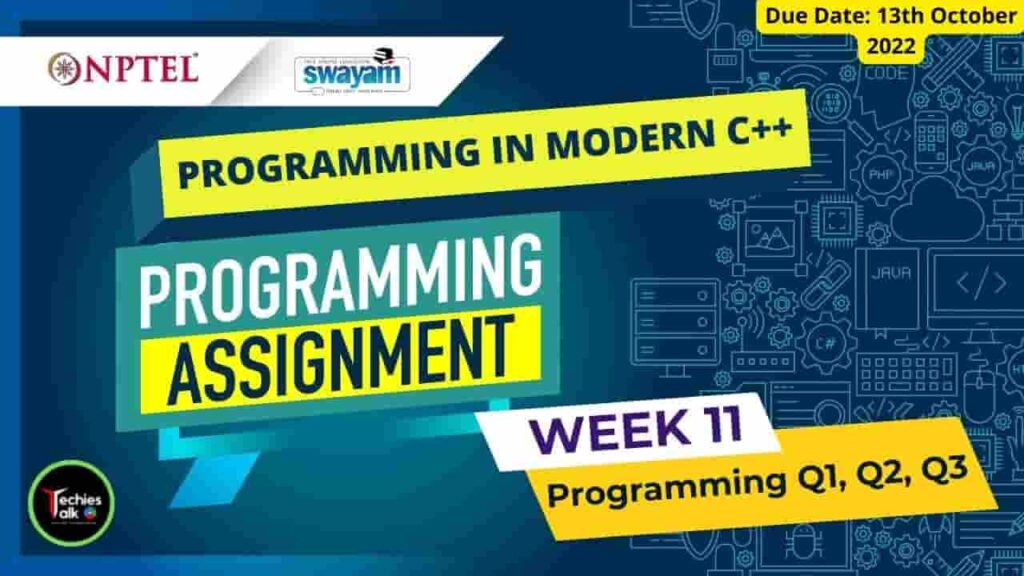
There has been a continual debate on which programming language/s to learn, to use. As the latest TIOBE Programming Community Index for August 2021 indicates – C (13%), Python (12%), C++ (7%), Java (10%), and C#(5%) together control nearly half the programming activities worldwide. Further, C Programming Language Family (C, C++, C#, Objective C etc.) dominate more than 25% of activities. Hence, learning C++ is important as one learns about the entire family, about Object-Oriented Programming and gets a solid foundation to also migrate to Java and Python as needed. C++ is the mother of most general purpose of languages. It is multi-paradigm encompassing procedural, object-oriented, generic, and even functional programming. C++ has primarily been the systems language till C++03 which punches efficiency of the code with the efficacy of OOP. Then, why should I learn it if my primary focus is on applications? This is where the recent updates of C++, namely, C++11 and several later offer excellent depths and flexibility for C++ that no language can match. These extensions attempt to alleviate some of the long-standing shortcomings for C++ including porous resource management, error-prone pointer handling, expression semantics, and better readability. The present course builds up on the knowledge of C programming and basic data structure (array, list, stack, queue etc.) to create a strong familiarity with C++98 / C++03. Besides the constructs, syntax and semantics of C++ (over C), we also focus on various idioms of C++ and attempt to go to depth with every C++ feature justifying and illustrating them with several examples and assignment problems. On the way, we illustrate various OOP concepts. The course also covers important advances in C++ 11 and later released features..
Programming In Modern C++ Week 11 Programming Assignment.
INTENDED AUDIENCE : Any interested audience
PREREQUISITES : 10th standard/high school
INDUSTRY SUPPORT : Programming in C++ is so fundamental that all companies dealing with systems as well as application development (including web, IoT, embedded systems) have a need for the same. These include – Microsoft, Samsung, Xerox, Yahoo, Oracle, Google, IBM, TCS, Infosys, Amazon, Flipkart, etc. This course would help industry developers to be up-to-date with the advances in C++ so that they can remain at the state-of-the-art.
Course Layout
Programming Assignment Q1
#include
class person{
public:
virtual void show() = 0;
};
class Employee : public person {
private:
double salary;
public:
Employee(const double& _salary) : salary(_salary){
std::cout << "lvalue" << " ";
}
Employee(double&& _salary) : salary(_salary){
std::cout << "rvalue" << " ";
}
void show(){ std::cout << salary << " "; }
};
class Player : public person {
private:
int rank;
public:
Player(const int& _rank) : rank(_rank){
std::cout << "lvalue" << " ";
}
Player(int&& _rank) : rank(_rank){
std::cout << "rvalue" << " ";
}
void show(){ std::cout << rank << " "; }
};
template //LINE-1
T createPerson(U&& s){ //LINE-2
T t(std::forward(s));
return t; //LINE-3
}
int main() {
double s;
int r;
std::cin >> s >> r;
auto p1 = createPerson(s);
auto p2 = createPerson(std::move(s));
auto p3 = createPerson(r);
auto p4 = createPerson(std::move(r));
std::cout << std::endl;
p1.show();
p2.show();
p3.show();
p4.show();
return 0;
}
Programming Assignment Q2
#include
template
class Data{
private:
T _d;
public:
Data(T data) : _d(data){}
T getData() const{
return _d;
}
};
template
struct Adder{
U operator()(std::ostream& os, Data&& d1, Data&& d2){
os << "rvalue version: ";
return (d1.getData() + d2.getData());
}
U operator()(std::ostream& os, const Data& d1, const Data& d2){
os << "lvalue version: ";
return (d1.getData() + d2.getData());
}
};
template //LINE-1
double apply(T& os, U param, V&& p1, W&& p2) { //LINE-2
return param(os, std::forward(p1),std::forward(p2)); //LINE-3
}
int main() {
int i;
double d;
std::cin >> i >> d;
Data d1(i);
Data d2(d);
std::cout << apply(std::cout, Adder(), d1, d2);
std::cout << std::endl;
std::cout << apply(std::cout, Adder(), std::move(d1), std::move(d2));
return 0;
}
Programming Assignment Q3
#include
#include
#include
int main(){
std::function,int)> RecMin; //LINE-1
RecMin = [&RecMin](std::vector tVec,int n)-> int{ //LINE-2
return n == 1 ? tVec[0] : tVec[n - 1] < RecMin(tVec, n - 1) ?
tVec[n - 1] : RecMin(tVec, n - 1);
};
auto Print = [&RecMin](std::vector tVec) {
std::cout << RecMin(tVec, tVec.size());
};
int n, m;
std::vector vec;
std::cin >> n;
for(int i = 0; i < n; i++){
std::cin >> m;
vec.push_back(m);
}
Print(vec);
return 0;
}